Chpater-2: Angular Fundamentals Part 2.
Now lets cover around 24 fundamental questions about Angular Robust Web framework.
Question: What is AOT in Angular?
Answer: In Angular, AOT stands for Ahead-of-Time compilation. This is a significant feature of Angular that compiles your application's HTML and TypeScript code into efficient JavaScript code during the build phase before the browser downloads and runs that code.
Essentially, AOT improves the performance of your applications in a couple of ways. First, it provides faster rendering by converting Angular HTML and TypeScript code into efficient JavaScript code during the build process, rather than at runtime. This means that the browser can execute the application immediately, without needing to wait for the Angular compiler to process the application code. Second, it results in smaller Angular framework download size because the browser no longer needs the compiler. The compiler is roughly half of Angular's size, so not having to send it to the client can significantly reduce the load time.Moreover, AOT improves security by compiling HTML templates and components into JavaScript files long before they are served to the client. With no templates to read and no risky client-side HTML or JavaScript evaluation, there are fewer opportunities for injection attacks.
Question: What is the reason for using the AOT compiler in Angular?
Answer: When we're talking about Angular's Ahead-of-Time (AOT) compiler, we're really focusing on performance and security. Unlike a Just-in-Time (JIT) compiler which compiles your app in the browser at runtime, the AOT compiler does this at build time.
So, what's the real-world benefit of this? Well, first up, it makes your app faster. Because the HTML and TypeScript code is converted to efficient JavaScript during the build process, your browser loads executable code which kicks into action much quicker. This means your app's rendering engine can get straight to work, providing a noticeably snappier experience for the user.Then there's the reduced size. Since the compiler doesn’t need to be downloaded with the app, that saves on the bandwidth, and users can start using your app much sooner.Let's not forget security. By compiling HTML templates and components before they run in the browser, AOT can catch errors early, and it also makes it difficult for malicious content to be injected into the app.
Question: What are the biggest advantages of AOT in Angular?
Answer: In a face-to-face Angular interview, when asked about the advantages of Ahead-of-Time (AOT) compilation in Angular, I would explain it in a concise and practical manner.
Firstly, AOT improves the performance of Angular applications. Unlike Just-in-Time (JIT) compilation, which compiles the application at runtime in the browser, AOT compiles the application during the build process.
This results in faster rendering as the browser loads pre-compiled HTML and JavaScript. Here's a basic contrast:
- JIT: Compiles the app in the browser, at runtime.
- AOT: Compiles the app during the build phase.
For instance, in an Angular CLI project, when you run ‘ng build --prod’, it automatically uses AOT compilation. Secondly, AOT enhances security. By compiling HTML templates and components into JavaScript files before they are served to the client, AOT eliminates the need for the browser to compile the code, which reduces the possibility of injection attacks.Lastly, AOT helps with template binding errors. It checks your templates during the build process, catching errors that would otherwise be caught at runtime in JIT.
This early detection of errors can significantly streamline development and debugging. In summary, the main benefits of AOT in Angular are:
- Performance boost: Faster rendering and reduced download size.
- Security improvement: Reduced risk of injection attacks.
- Error detection: Early detection of template binding errors.
Question: What is JIT in Angular?
Answer: Just-In-Time (JIT) compilation is a type of Angular compilation that happens at runtime, in the browser. Unlike Ahead-of-Time (AOT) compilation, which happens during the build process, JIT compilation translates your application's templates and components into JavaScript that the browser can interpret on the fly, as your application loads.
Here's why that matters: during development, JIT compilation allows for a faster development cycle because it skips the AOT compilation step. This means that when you make changes to your code, you can see those changes reflected in the browser almost immediately after refreshing.
However, JIT compilation can result in slower app startup times in production because the browser has to do more work at runtime. This is why for production builds, Angular applications typically use AOT compilation instead.To give you an example, when you bootstrap an Angular application with ‘platformBrowserDynamic().bootstrapModule(AppModule)’, you're using JIT compilation. Here's a snippet of code that you might use in a main.
ts file for a JIT-compiled application:

Question: What is the scope hierarchy in Angular?
Answer: In Angular, the scope hierarchy is designed around a tree structure where each Angular component may have its own scope, but can also inherit scope from its parent, creating a hierarchy of scopes. This hierarchy ensures that the application state can be managed effectively, allowing child components to be isolated when necessary, or to share state through inherited scopes. For example, in Angular, we typically have a root module that bootstraps our application. Within this module, we can have multiple components, each with its own scope. A parent component can pass down data to its children through property bindings, and children can communicate back to the parent using event emitters.
Here's a simple code example to illustrate how data can flow from a parent component to a child component:
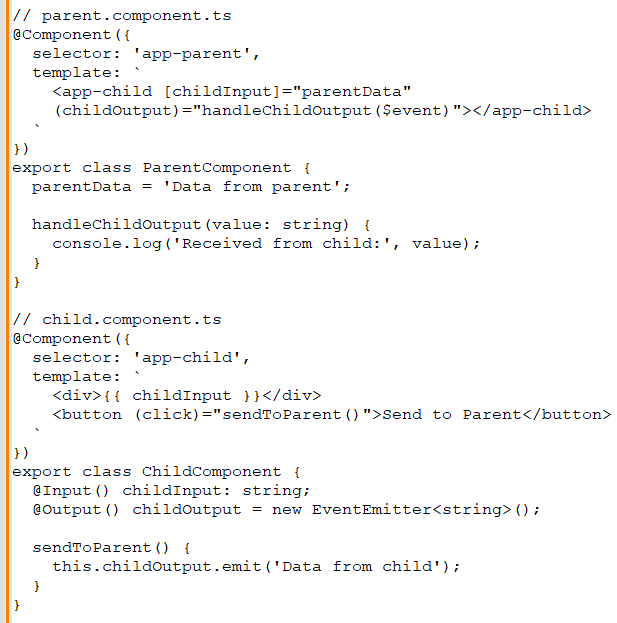
Question: What are the main building blocks of an Angular application?
Answer: In Angular, the main building blocks are Modules, Components, Templates, Metadata, Data Binding, Directives, Services, and Dependency Injection. Let me break it down:
Modules: Angular apps are modular and Angular has its own modularity system called NgModules. Every Angular app has at least one module, the root module, conventionally named ‘AppModule’. It provides the bootstrap mechanism that launches the application.
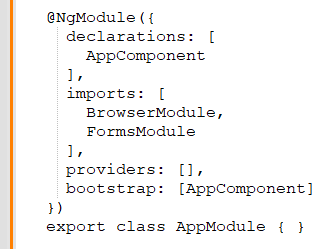
Components: They are the fundamental building blocks of Angular applications. A component controls a patch of screen called a view. For example, you could have a ‘LoginComponent’ controlling a part of the screen for logging in.
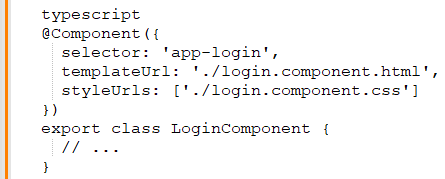
Templates: This is the HTML part that tells Angular how to render the component. It’s where you put your HTML enhanced with Angular’s template syntax.
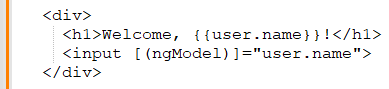
Metadata: Metadata tells Angular how to process a class. For instance, what is a component, what are its inputs and outputs, etc.
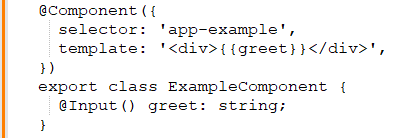
Data Binding: It’s the mechanism to coordinate the parts of your template with the parts of your component. Data binding can be one-way or two-way, binding from the component to the template, and vice-versa.
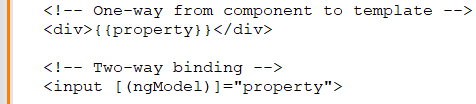
Directives: They are classes that add additional behavior to elements in your Angular applications. With Angular's built-in directives, you can manage forms, lists, styles, and what users see.
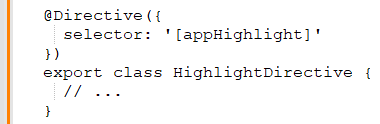
Services: They are a wide category encompassing any value, function, or feature that an app needs. A service is typically a class with a narrow, well-defined purpose. It should do something specific and do it well.
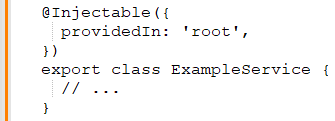
Dependency Injection (DI): It’s a design pattern and a core mechanism in Angular. DI lets you inject services into components, making your code more modular, reusable, and efficient.

Question: What is the difference between Observables and Promises in Angular?
Answer: When we talk about Observables and Promises in Angular, we're delving into the realm of asynchronous programming. Let's break it down in a more conversational way.
Promises are a part of JavaScript, and they represent a value that may be available now, later, or never. They're like saying, "I promise to return something to you. " The key with a Promise is that it will only return a single value and it's not cancellable. You can think of it as a one-time deal.
For example, if you have a function that returns a Promise, it might look something like this:
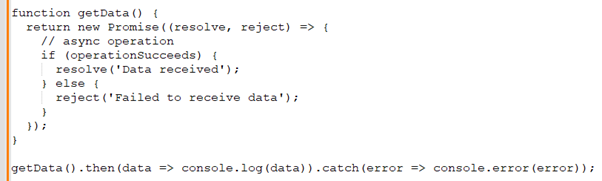
Here’s how you might set up an Observable:
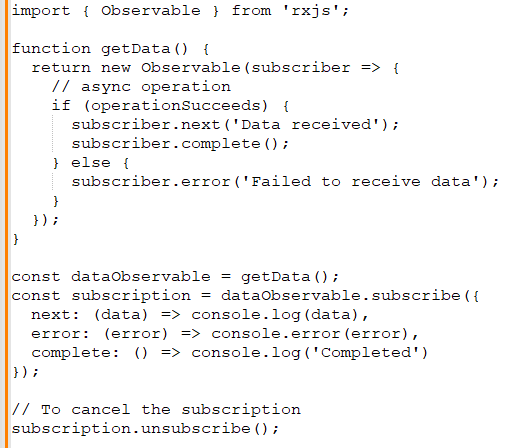
Question: What are directives in Angular?
Answer: In Angular, directives are a fundamental feature that allow you to attach behavior to elements in the DOM. Essentially, they are classes with the ‘@Directive’ decorator that can manipulate the behavior, appearance, or structure of a DOM element. There are three types of directives in Angular:
Components - These are directives with templates. They're the most common kind of directive, and when you create a new component, it's actually an Angular directive with a view.Structural Directives - These directives change the DOM layout by adding and removing DOM elements. For example, ‘*ngFor’ and ‘*ngIf’ are structural directives. When you see an asterisk (*) before a directive, it's a sign that it's structural.
Here’s a simple code example using ‘*ngIf’:

Let’s look at an example using ‘ngStyle’:

Question: What is lazy loading in Angular?
Answer: In Angular, lazy loading is a design pattern that's all about speed and efficiency. It's like when you're watching a movie online, and you know how it loads just the part you're watching, not the whole thing at once? Lazy loading in Angular is similar. It means that certain parts of your app, like components or modules, are only loaded when they're needed, usually when a user navigates to a particular route. This is great because it reduces the initial load time of the app. Your users don't have to wait for everything to load before they can start using the app. Instead, they get to the starting line faster, and the rest of the app loads in the background or as they need it. Here's a quick code example to show how it works.
Normally, in your Angular routes, you might have something like this:

But with lazy loading, you set it up like this:

Question: What is Angular Router?
Answer: In an Angular context, "opinionated architecture" refers to a framework or set of tools that provides clear guidance on how applications should be structured and how various problems should be approached. Angular is often described as opinionated because it encourages a specific structure and set of patterns for building applications. Angular enforces a certain level of uniformity by prescribing how components should communicate, how data should flow through an application, and how services should be implemented. This is beneficial because it leads to consistency across different Angular projects, making it easier for developers to understand and contribute to a new Angular application if they are already familiar with the framework. For instance, Angular has a specific way of handling client-side navigation through its Router. This service allows you to define routes in your application and associate them with components. When a user navigates to a particular URL, the Router loads the corresponding component and renders it. It also provides features like route guards for controlling access to certain routes, and resolvers for pre-fetching data before a route is activated.
Here’s a basic example of how to define routes in an Angular application:
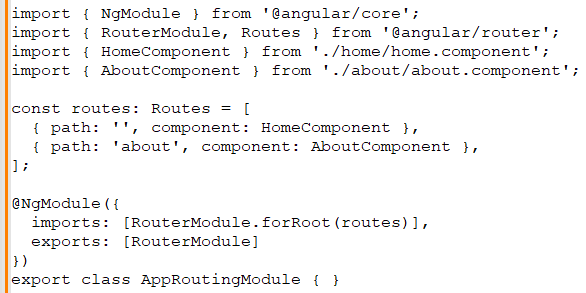
In this code snippet, we're defining two routes: a default route that will render ‘HomeComponent’ when the app loads, and an ‘/about’ route that will render ‘AboutComponent’. This kind of declarative routing is part of what makes Angular opinionated; it has a specific way it wants you to handle navigation, which is integrated into its overall architecture.
Question: What do you mean by the router imports?
Answer: In Angular, the term "router imports" refers to the mechanism by which we import the ‘RouterModule’ into our application, usually within a feature module or the root module, to configure the routes. This is fundamental for enabling navigation between different components based on the URL path accessed in the browser.
For example, if we have an application with a homepage, an about page, and a contact page, we would configure our routes in an array with objects defining the path and the component that should be rendered. Here's a simplified code snippet to illustrate this:
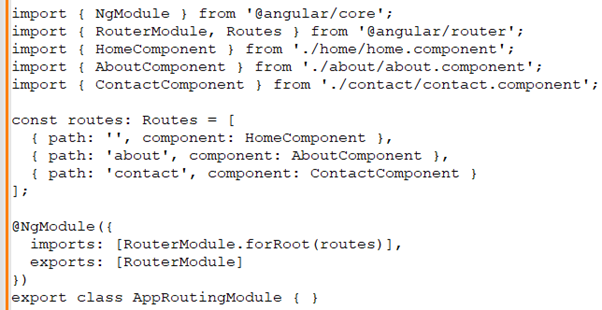
Question: What do you mean by RouterOutlet and RouterLink?
Answer: RouterOutlet and RouterLink are part of Angular's routing mechanism which allows you to navigate between different components in your application.
RouterOutlet is a directive from the RouterModule that acts as a placeholder that Angular dynamically fills based on the current router state. It's where the content of your routed component will be displayed.
Think of it like a stage in a theater — it's the spot where the current component is showcased depending on which route is active. For example, in your app's template, you might have:

RouterLink, on the other hand, is a directive that you use to link to specific routes. It's similar to the traditional anchor tag , but it works within the confines of the Angular app to navigate without refreshing the page. Here's how you might use it in your templates:

Question: What are the different router events used in Angular Router?
Answer: In Angular, the router provides several navigation events that we can listen to for tracking routing changes and the lifecycle of route navigation. These events are a part of the router's event stream and can be quite handy for things like loading indicators, logging, or analytics. Here are the key router events:
- NavigationStart: This event is fired when navigation starts.
- RouteConfigLoadStart: When the router starts to load a route configuration lazily, this event is fired.
- RouteConfigLoadEnd: This event is fired when a route configuration has finished loading.
- RoutesRecognized: This event is triggered when the router has parsed the URL and recognized what route these belong to.
- GuardsCheckStart: This event occurs when the router starts the guards phase of routing.
- ChildActivationStart: This event is triggered when the router begins activating a route's children.
- ActivationStart: When the router begins activating a route, this event is fired.
- GuardsCheckEnd: This event is emitted when the router has completed the guards phase of routing.
- ResolveStart: This event is triggered when the router begins the resolve phase of routing.
- ResolveEnd: This event is fired when the router completes the resolve phase of routing.
- ActivationEnd: After the router activates a route, it emits this event.
- ChildActivationEnd: This event is fired after the router has activated all the children of a route.
- NavigationEnd: This event is emitted when navigation ends successfully.
- NavigationCancel: If navigation is canceled, this event is emitted, often due to a route guard returning false.
- NavigationError: When navigation fails due to an unexpected error, this event is emitted.
- Scroll: This event is fired when the router scrolls to an element on the navigation path.
To listen to these events, you would inject the ‘Router’ service and subscribe to its events property, like so:
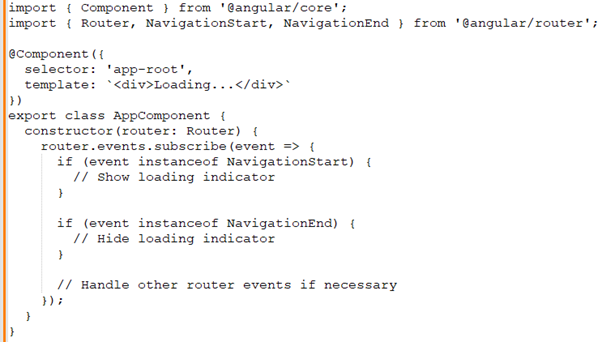
Question: What do you mean by the RouterLinkActive?
Answer: In Angular, ‘RouterLinkActive’ is a directive that you can use on an element, such as a link, to track whether a route's link is active or not. When the link is active, Angular will apply a CSS class to the element, which is by default 'active', but you can customize it.
The real benefit of ‘RouterLinkActive’ is in its dynamic nature. It responds to changes in the active state of its associated ‘RouterLink’ without you having to manage the state manually. This is particularly useful for things like navigation menus, where you want to visually indicate which section of the application the user is currently viewing.
For example, if you have a navigation bar with links to different components in your app, you can use ‘RouterLinkActive’ to highlight the current page:

Question: What do you mean by the RouterState?
Answer: In the context of Angular, the RouterState is essentially a representation of the state of the router at a moment in time. It's a tree of activated routes, where each route contains various information like the path and the parameters. When you navigate in an Angular application, the router updates this state accordingly. For instance, if your Angular application has multiple routes and you navigate from one to another, the RouterState will change to reflect the new route. It's useful because it helps you track where you are in your application, manage data fetching, and understand the hierarchy of your routing structure.
If you wanted to access the current RouterState within your component, you could inject the ‘Router’ service and use the ‘routerState’ property. Here's a quick example:
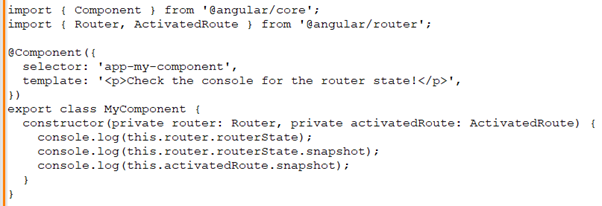
Question: What is HttpClient, and what are the advantages of it?
Answer: In Angular, ‘HttpClient’ is a built-in service provided by the ‘@angular/common/http’ module, which offers a simplified client HTTP API for Angular applications that rests on the XMLHttpRequest interface exposed by browsers. It's a more powerful and flexible way to handle HTTP requests compared to the older ‘Http’ service that was part of Angular's earlier versions.
The advantages of using ‘HttpClient’ include:
Interceptors: You can declare interceptors that allow you to catch and handle requests or responses globally. This is extremely useful for tasks like adding headers, logging requests, or handling errors uniformly across your application.
Observable-based: ‘HttpClient’ methods return RxJS Observables by default, unlike the old ‘Http’ service which returned Promises. This gives you a robust set of operators to handle asynchronous operations more efficiently, like retrying failed requests, transforming data, and cancelling requests.
Typed Response: You can enforce type checking in your HTTP responses, which can greatly reduce errors and provide a better development experience with strong typing.
Simplified Syntax: It simplifies the syntax for making HTTP requests and handling responses, reducing the boilerplate code you need to write.
Testing: It is easier to test than the previous ‘Http’ service because you can use the ‘HttpClientTestingModule’ and ‘HttpTestingController’ for mocking and flushing requests in your tests.
Here's a quick code example that shows how to use ‘HttpClient’ to make a GET request:
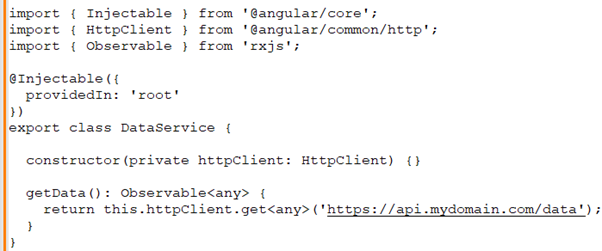
Question: Can Angular applications be configured to perform server-side rendering instead of the default client-side rendering?
Answer: Absolutely, Angular applications can indeed be configured for server-side rendering (SSR). This is typically achieved using a technology called Angular Universal. What Angular Universal does is, it allows Angular apps to be rendered on the server side, which is a fantastic solution for SEO purposes and for improving the load time of the application on the client side.
Here’s how it works: When a user requests a page, the server quickly renders and serves the application in a fully rendered state, which is immediately viewable by the user. It doesn’t need to wait for all the JavaScript to kick in to become interactive. This is particularly beneficial for users on slower networks or devices. The Angular CLI even provides support to add SSR to your project with a simple command like ‘ng add @nguniversal/express-engine’. This sets up the necessary configurations. Once the server-side app is built with the ‘ng run’ command, it can be served with Node.js, and your Angular app will be rendered from the server. To give you an example, when you serve an Angular app with SSR, you have a server file, typically ‘server.ts’, which sets up Express to listen for incoming requests and return the rendered application.
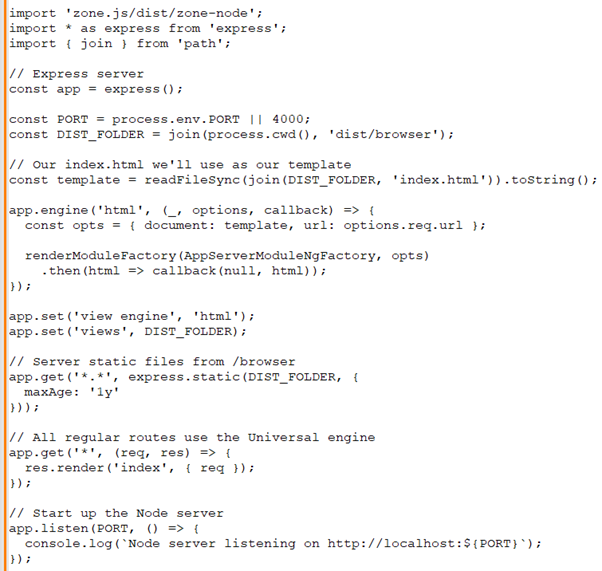
Question: How should one effectively manage and implement error handling within an Angular application?
Answer: In an Angular application, effective error handling is critical for maintaining a smooth user experience and for debugging during development. My approach to this involves a few key strategies:
Use Angular’s built-in ErrorHandler: By default, Angular provides an ErrorHandler class that can be used to create a custom error handler service. This allows for global error handling throughout the application.
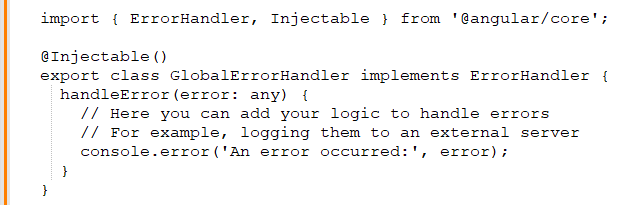
Then, you provide it in your app module:

HTTP Interceptors for API calls: When making HTTP requests, use interceptors to catch and handle HTTP errors in a centralized way.
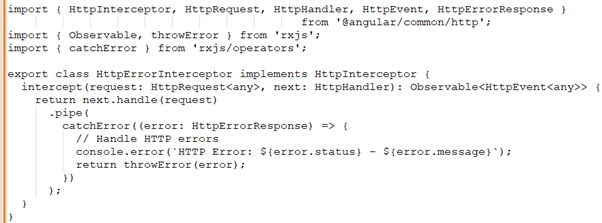
Component-level error handling: Within components, especially when dealing with user-driven events, try-catch blocks can be used to handle errors that may occur during the execution of specific code blocks.
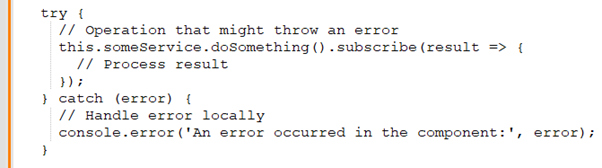
User notifications: For a better user experience, it’s important to inform users when an error occurs. This can be done using a toast notification service or a modal dialog, depending on the severity of the error.
Logging: Implement a logging service to send errors to a backend system for analysis. This helps in identifying patterns in errors and resolving them in future releases.
Testing: Finally, thoroughly test error handling logic using Angular’s testing frameworks to ensure that it behaves as expected.
Effective error handling is as much about anticipating and managing errors as it is about responding to them. It should aim to provide feedback to the user, offer a path forward whenever possible, and log diagnostic information for developers to analyze. This leads to a more resilient and user-friendly application.
Question: Could you explain the process of initializing an Angular application, often referred to as bootstrapping?
Answer: Absolutely, bootstrapping in Angular refers to the process of initializing and loading an application. When we talk about bootstrapping an Angular app, we're essentially discussing the steps Angular goes through to start up the application.
It begins when we call the ‘bootstrapModule’ method on the platform that we're targeting, typically the browser.
This method takes an Angular ‘@NgModule’ as a parameter, which serves as the root module for the application. Here's an example of bootstrapping an app using the ‘AppModule’:

Question: What is the digest cycle process in Angular?
Answer: In AngularJS, the digest cycle is a core part of the framework that's responsible for monitoring the watchlist for changes and updating the DOM to reflect these changes. This process is what makes two-way data binding possible in AngularJS.
When a watch is set up, AngularJS creates a watcher object that looks at the value it's supposed to keep an eye on. Each time a piece of code that could potentially change model data is executed, such as a controller function triggered by a user event, AngularJS enters a digest cycle.The cycle goes through all the watchers and checks if any watched values have changed. If a change is detected, the watcher's listener function is called, which typically updates the DOM with the new value. This is known as a "dirty check."After the first pass, AngularJS checks again for changes, because the listeners might have caused more changes. This cycle repeats until no more changes are detected or a maximum number of iterations is reached, to prevent an infinite loop.
Here's a simplified code example to illustrate how you might manually trigger a digest cycle in AngularJS:

Question: In Angular, what distinguishes a Component from a Directive in terms of functionality and use?
Answer: In Angular, the distinction between a Component and a Directive is fundamental to its architecture and how the framework manages the Document Object Model (DOM).
A Component in Angular is essentially a Directive with a template. It's the most basic UI building block of an Angular application. A component controls a patch of screen called a view through its associated template, which defines a segment of the HTML to render, and the component class, which contains the logic behind the view. For example, you could have a ‘UserProfileComponent’ that has its own HTML template and accompanying class file that contains the data and behaviors related to a user profile.
Here's a simple example of a component:
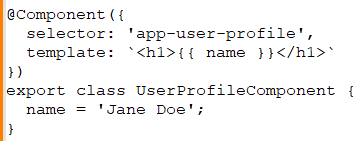
A Directive, on the other hand, is a way to add behavior to elements in the DOM. Unlike components, directives don't have their own views and typically modify or extend the behavior of existing elements. There are two types of directives in Angular aside from components: Structural directives that change the DOM layout by adding and removing DOM elements (like ‘*ngFor’ or ‘*ngIf’), and Attribute directives that change the appearance or behavior of an element, component, or another directive (like ‘ngStyle’ or ‘ngClass’).
Here's an example of a simple attribute directive:
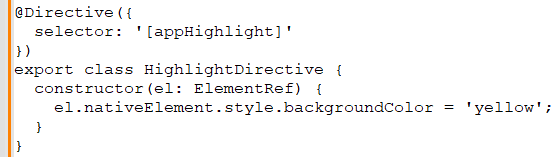
Question: How would you explain the MVVM architectural pattern as it is implemented in Angular?
Answer: In an interview setting, I would approach the explanation of the Model-View-ViewModel (MVVM) architectural pattern in Angular with a conversational tone and try to relate it to practical experiences.
MVVM is a structural design pattern that separates the development of the graphical user interface from the business logic or back-end logic.
In Angular, this pattern is manifested through its framework components:
Model: This represents the data and the business logic of the application. In Angular, it’s typically realized through services or classes that define the data structures and possibly methods to manipulate the data. It’s the pure data of the application, without any concern for how it’s presented to the user.
View: This is what the user interacts with. It's composed of the templates, which are HTML enhanced with Angular directives and bindings. The View is dumb in the sense that it doesn’t process any logic or maintain any state; it just presents the data provided by the ViewModel and sends user actions back to the ViewModel.
ViewModel: Angular brings this concept to life through its components and directives. The ViewModel is responsible for exposing the data and command objects that the View needs, and for managing the View’s presentation logic and state. This is where you write your handlers and presentation logic. For instance, if you have a user list, your Model would be the user objects, perhaps fetched from an API.
The ViewModel would be the Angular component that includes logic to handle user interactions and prepare data to display. The View would be the template that uses Angular’s data binding to display the user list. Here’s a small code snippet to illustrate this:
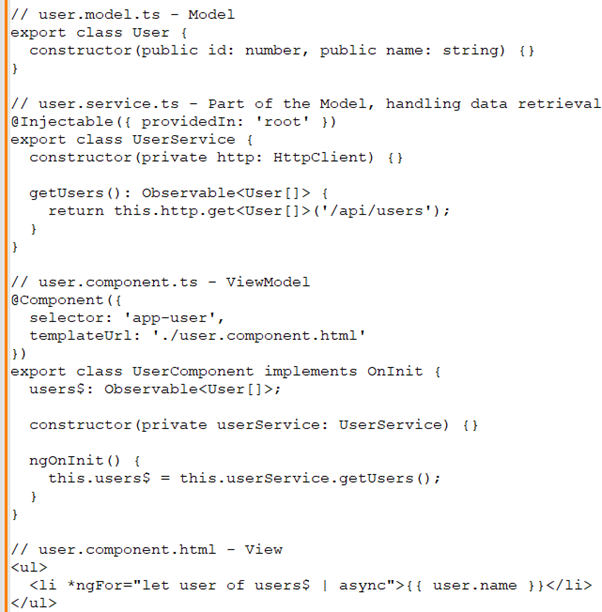
Question: How does AsyncPipe function within Angular, and what are its primary uses?
Answer: In an Angular context, the term "opinionated architecture" refers to a framework or environment that encourages or facilitates a certain way of doing things, often through built-in decisions that enforce particular patterns and practices. Angular is often considered an opinionated framework because it provides a set of conventions and default behaviors that guide developers on how to structure an application, how to manage data flow, how components should communicate, and how to handle various other aspects of application development. For instance, Angular encourages the use of TypeScript for better type safety and easier refactoring, provides a well-defined component lifecycle, enforces a particular module system, and has a strong opinion on how to manage state and dependencies through services and dependency injection. These conventions and guidelines aim to promote best practices, consistency, and maintainability, especially in large and complex applications. One of the real strengths of this approach is that it reduces the number of decisions that a developer needs to make, speeding up the development process. However, it also means that for projects that might not fit into these conventions, developers might find it more challenging to adapt the framework to their needs. It's a trade-off between the ease of getting started with best practices in place and the flexibility to deviate from those practices when the project calls for it.To give a practical example, when you generate a new Angular component using the Angular CLI, it automatically provides you with a TypeScript file for your component class, an HTML file for your template, a CSS file for styles, and a spec file for testing. This structure is an opinion that Angular has about how components should be organized. It's possible to deviate from this structure, but Angular's tooling and documentation all encourage you to follow this pattern.
ReadioBook.com