Chapter 5: Angular Advanced Interview Questions.
This chapter of the Angular Interview Questions ReadioBook delves into advanced Angular concepts that are frequently encountered in technical interviews. This chapter is designed to prepare you for in-depth discussions on more intricate aspects of Angular development.
Key Concepts Covered in this Chapter:
- Dependency Injection (DI): Explore advanced DI techniques, such as custom providers, scope hierarchies, and component lifecycle hooks.
- Routing: Master advanced routing concepts, including lazy loading, route guards, and custom route resolvers.
- Forms: Gain proficiency in handling complex forms, including form validation, form submission, and reactive forms.
- Testing: Uncover advanced testing strategies, including unit testing, integration testing, and end-to-end testing.
- Performance Optimization: Learn techniques to optimize Angular applications for performance, including change detection, lazy loading, and tree shaking.
Preparing for Advanced Angular Interview Questions:
- Review Fundamental Concepts: Ensure you have a solid understanding of core Angular concepts before diving into advanced topics.
- Practice with Examples: Work through real-world scenarios and examples to solidify your understanding of advanced Angular concepts.
- Explore Open-Source Projects: Examine the code of open-source Angular projects to see how advanced concepts are applied in practice.
- Engage with the Angular Community: Participate in online forums and communities to seek guidance and insights from experienced Angular developers.
Benefits of Mastering Advanced Angular Concepts:
- Enhanced Problem-Solving Skills: Tackle complex Angular challenges with confidence and creativity.
- Increased Job Opportunities: Stand out from the competition and attract top-tier Angular development positions.
- Greater Project Impact: Deliver high-performance, scalable, and maintainable Angular applications.
- Career Advancement: Position yourself for leadership roles and career growth in the Angular ecosystem.
By mastering the advanced Angular concepts covered in this Chapter, you'll elevate your skills to an expert level, empowering you to tackle the most demanding Angular challenges and excel in your career journey. So now lets start.
Question: Can you please give some detail about MongoDB?
Answer: MongoDB is a NoSQL database that's often used in modern web applications, including those built with Angular. Angular is a front-end framework that deals with the user interface and user interactions, while MongoDB is typically used on the server-side to store and retrieve data.
In an Angular application, you wouldn't interact with MongoDB directly from the front-end. Instead, you'd have an API layer in between, often built with Java SpringBoot, Node.js and the Express framework. This API layer is responsible for handling HTTP requests from the Angular application, performing operations on the MongoDB database, and sending responses back to Angular.Let's say we have an Angular service that needs to fetch user data from a MongoDB database.
Here's a basic example of how that might look:
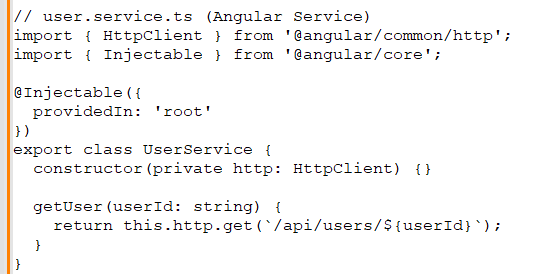
On the server side, you might have an Express route that handles the request:
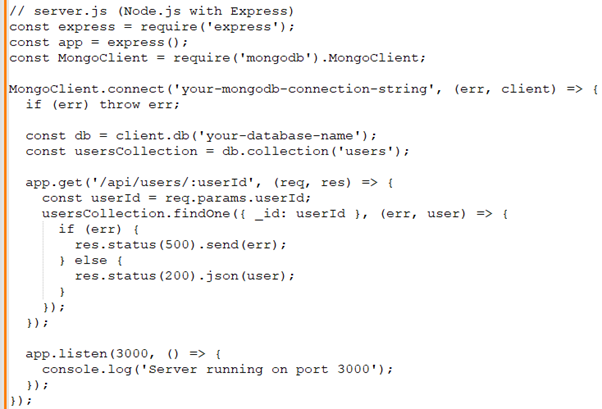
Question: What is BSON format?
Answer: BSON stands for Binary JSON, and it's essentially a binary-encoded serialization of JSON-like documents. It's designed to be efficient in both space and speed, so it's often used in scenarios where performance is critical, such as database operations.
For example, MongoDB uses BSON to store documents. BSON extends the JSON model to provide additional data types, such as Date and binary data types, and to be efficient for encoding and decoding within different languages.
Here’s a quick example in JavaScript, which you might use in a Node.js application interfacing with MongoDB:
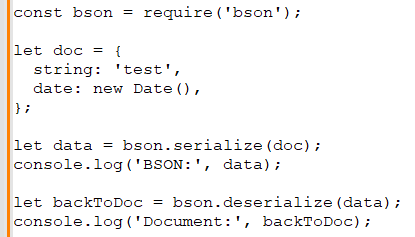
Question: What MongoDB compass?
Answer: MongoDB Compass is an official graphical user interface (GUI) tool provided by MongoDB. It allows you to manage your MongoDB database through a rich and intuitive interface. With Compass, you can query your data, run aggregation pipelines, view database schema, inspect indices, perform CRUD (Create, Read, Update, Delete) operations, and much more without needing to use the command line. One of the key features of Compass is its schema visualization, which allows you to understand the structure of your data and identify any irregularities. It also provides performance insights by allowing you to analyze query performance and optimize your database with visual explain plans.
Question: What are Validators?
Answer: In Angular, ‘Validators’ are part of the ‘@angular/forms’ package and are used to perform validation on form controls, providing error messages when the data entered by a user does not meet specific criteria set for a form field. Validators can be applied to both template-driven and reactive forms. For instance, if you have a form control for an email address, you can attach the ‘Validators.required’ and ‘Validators.email’ validators to ensure that the field is not left blank and that the entered text is a valid email address.
Here's a code example for a simple reactive form with validators in a component:
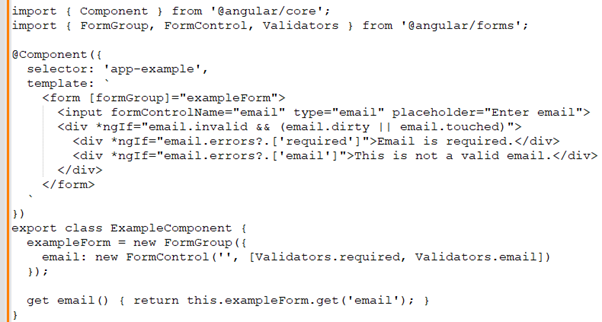
Question: What is FormGroups?
Answer: FormGroups in Angular are a fundamental concept in reactive forms that allow you to track the value and validation status of a group of FormControl instances. A FormGroup instance aggregates the values of each child FormControl into one object, with each control name as the key. It's like a form model that you create in your component class, rather than with directives in your template.
Let's say you have a form where you need the user to input their first name and last name. In your Angular component, you would create a FormGroup like this:
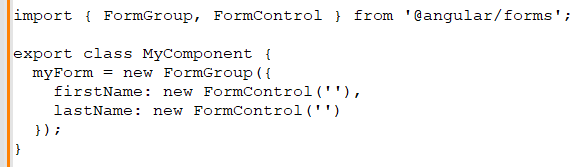
Question: What is FormControl?
Answer: FormControl is a fundamental building block in Angular's reactive forms, which is part of the larger FormGroup. It tracks the value and validation status of an individual form control, like an input field. FormControl offers the main API for interacting with a single control, providing properties and methods for handling data binding, validation, and overall state.
For instance, when you're creating a simple login form with a username field, you would instantiate a FormControl to keep track of the username input. Here's a quick example:

Question: What is reactive form or template approach?
Answer: In Angular, forms are a key part of many applications, and there are two approaches to managing form data: reactive forms and the template-driven approach.
The reactive form approach is more scalable, robust, and testable. It is favored when you have complex form interactions and validation requirements. Reactive forms provide you with model-driven forms, where you create the form structure in the component class. By using reactive forms, you create a form group instance manually and control the form model directly. You define the structure of the form as well as the validation in the component class itself.
Here's a basic example of a reactive form with a single input field with validation in Angular:
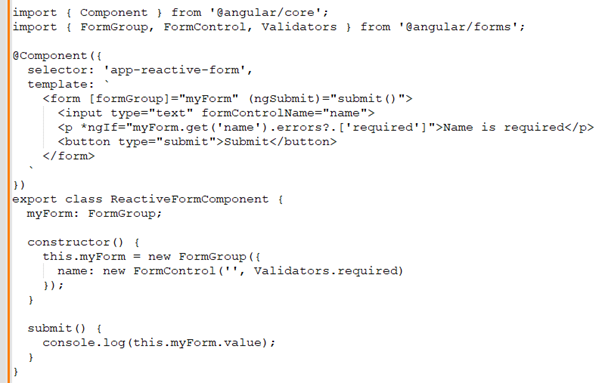
Question: What is NodeJS?
Answer: Node.js is a runtime environment for executing JavaScript code outside of a browser. It's built on the V8 JavaScript engine from Google, which is the same one used in Google Chrome, allowing Node.js to provide a very efficient way to build scalable network applications.
Node.js is event-driven and uses non-blocking I/O, which makes it lightweight and efficient, especially for data-intensive real-time applications that run across distributed devices.
It's also used for building backend services like APIs and handling web requests. Here's a simple example of creating a web server in Node.js:
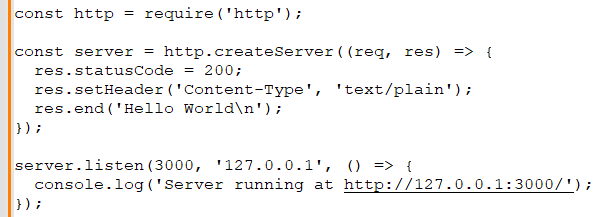
Question: What is Express?
Answer: Express, also known as Express.js, is a web application framework for Node.js. It's designed to build web applications and APIs with a focus on simplicity and minimalism. It provides a robust set of features to develop both web and mobile applications. Express streamlines the server creation process for Node.
js by handling many of the tedious tasks involved, such as routing and request handling. Let me give you an example of setting up a simple server with Express:
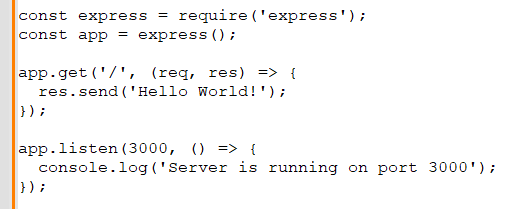
Question: What is Mongoose?
Answer: Mongoose is an Object Data Modeling (ODM) library for MongoDB and Node.js. It manages relationships between data, provides schema validation, and is used to translate between objects in code and the representation of those objects in MongoDB. For example, suppose we have a simple User model. Using Mongoose, we would define it like this:
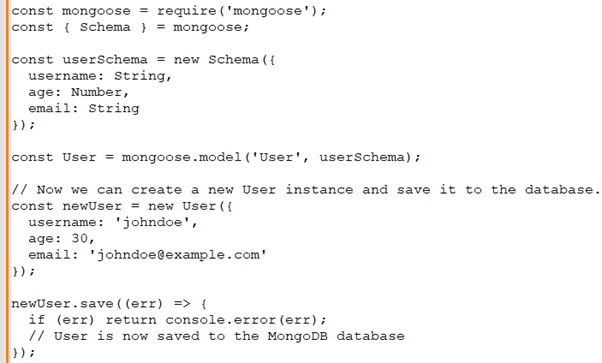
Question: What is body-parser?
Answer: Body-parser is a middleware that was commonly used with Express applications in Node.js. Its primary purpose is to parse incoming request bodies before your handlers, available under the ‘req.body’ property. It is particularly useful when you want to read HTTP POST data.
In earlier versions of Express, body-parser was not bundled with Express and had to be installed separately.
However, with newer versions, its functionality is built directly into Express. Here's a simple code example of using body-parser in an Express app to parse JSON bodies:
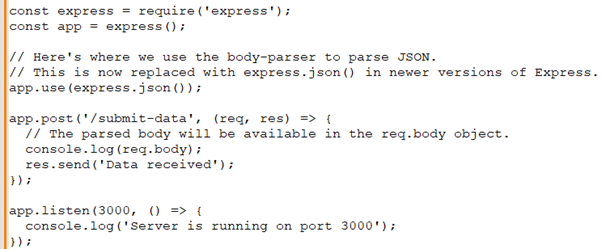
Question: What is collection in MongoDB?
Answer: In MongoDB, a collection is quite similar to a table in the traditional relational database management systems (RDBMS). It's where documents are stored, and these documents are the records or rows equivalent in RDBMS. However, unlike a table, a collection does not enforce a schema. This means that the documents within a single collection can have different fields from each other.
For instance, in an Angular application where you're working with a MongoDB database, you might have a service that interacts with a collection. Here's a basic example in TypeScript using the MongoDB Node.js driver:
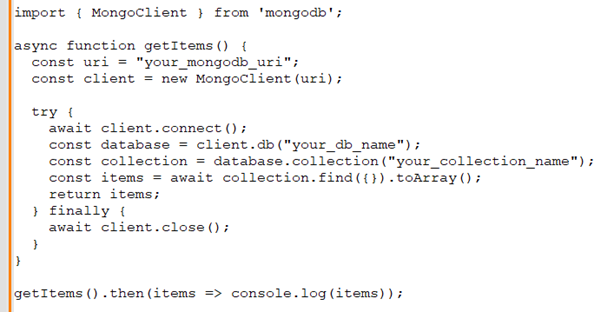
Question: What is testing in Angular app?
Answer: Testing in an Angular application refers to the process of checking that the different parts of the app work as expected. Angular provides a robust environment for testing components, services, and other classes through unit tests, which test pieces in isolation, and end-to-end tests, which test the behavior of the application as a whole.
In Angular, we typically use Jasmine for writing test specs and Karma for running the tests. For example, if we have a simple component that includes a method to increment a value, a unit test in Jasmine would look like this:
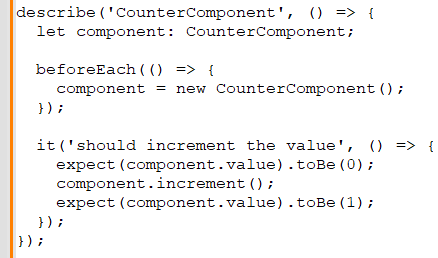
Question: What is build suits in Angular app testing?
Answer: Build suits in Angular are essentially collections of related tests that are intended to be executed together. When it comes to Angular applications, tests are typically divided into unit tests and end-to-end (E2E) tests. The unit tests focus on small, isolated pieces of code, such as components, services, and pipes, while end-to-end tests are used to test the application as a whole, simulating user interaction with the app.
Angular provides the Karma test runner for unit testing, which allows you to define a suite of tests using the ‘describe’ function. Within each suite, you use the ‘it’ function to define individual test cases. Here's a simple example of a test suite in an Angular application:
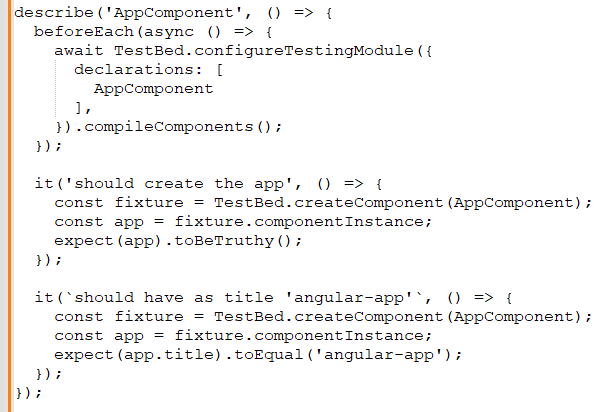
Question: What is Jasmin and why we use it?
Answer: Jasmine is a behavior-driven development framework for testing JavaScript code. It does not rely on browsers, DOM, or any JavaScript framework. Thus, it's suited for websites, Node.js projects, or anywhere that JavaScript can run.
It allows one to write tests in a clean and readable format that not only tests the functionality but also serves as documentation.
The syntax is simple, and it comes with a lot of features out of the box, like test doubles and asynchronous testing support. Here’s a basic example of a Jasmine test suite with a single test:
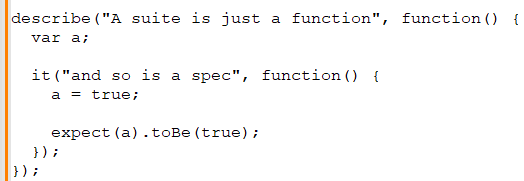
Question: What is DOM?
Answer: DOM stands for Document Object Model, which is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as a tree of nodes and objects; this way, programming languages can interact with the page.
A simple example of using the DOM in JavaScript might look like this:

Question: What is Karma?
Answer: Karma is a test runner created by the AngularJS team that is designed to work with any framework. It allows you to execute tests in real browsers, which is essential for capturing all the nuances of user interactions in different environments. Karma also integrates with popular continuous integration tools, making it easier to incorporate into your development workflow.
Here's a simple example of how you might set up a test using Karma with Jasmine, a popular testing framework:
First, you install Karma and the necessary plugins:

Then, you create a ‘karma.conf.js’ configuration file:
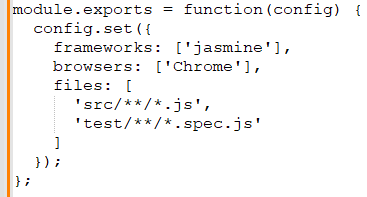
The ‘files’ array specifies the paths to the source files and the test files. To run tests, you simply execute:
karma start.Karma will launch Chrome, execute the tests specified in your test files, and output the results to the terminal. It's a very hands-on tool that fits right into the iterative nature of testing during development. Karma is a test runner developed by the Angular team, designed to automate tasks related to the execution of the developer tests with Jasmine.
Question: What is specs?
Answer: In the context of Angular and software development in general, "specs" typically refers to specifications, which are detailed descriptions of the requirements, design, behavior, or functionality of an application. In the case of Angular, specs are often associated with testing, particularly unit testing. Specs describe the expected behavior of the application's components, services, directives, etc.
In Angular, specs are written using a testing framework like Jasmine or Mocha. They are used to define test cases that validate whether different parts of the application are working as intended. Here's an example of a simple spec in Jasmine that tests a component in Angular:
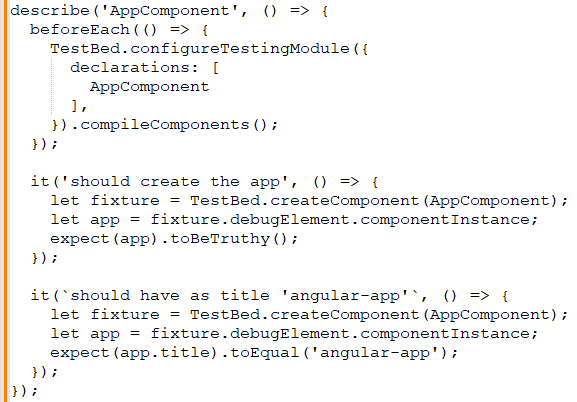
Inside this suite, we have two specs defined with ‘it()’: one checks that the component is created, and the other checks that the component's title property is initialized as expected. Specs are a crucial part of test-driven development (TDD) and behavior-driven development (BDD) methodologies, where they guide the development process by specifying the criteria that the code must meet to be considered complete.
Question: What is BDD and TDD?
Answer: Behavior-Driven Development (BDD) and Test-Driven Development (TDD) are both methodologies used in software development focused on ensuring the implementation of functional software that meets requirements.
TDD is a development approach where the developer writes a test before writing just enough production code to fulfill that test and refactoring.
The process starts with designing and developing tests for every small functionality of an application. A simple cycle of TDD is as follows:
- Add a test.- Run all tests and see if the new test fails.- Write the code.- Run tests.- Refactor code.- Repeat.
In Angular, this could mean starting with a test for a component or service. For example, if you have a service to fetch user data, you'd start by writing a test case for the expected behavior:
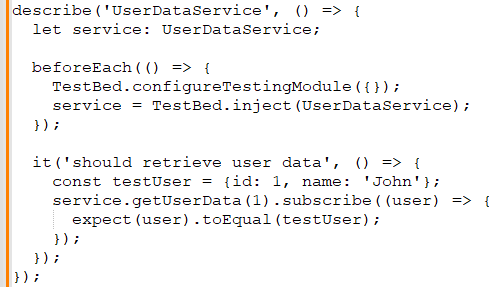
Here's an example of a BDD scenario:
Feature: User profile.
Scenario: Viewing user data.
Given the user has logged in. When the user navigates to their profile page. Then they should see their name and email.The above scenario would then be backed by step definitions that tie your human-readable language to code execution. In practice, BDD and TDD can intersect, especially in a framework like Angular, where testing is encouraged and facilitated. BDD can be seen as a high-level layer on top of TDD practices.
Question: What is describe method?
Answer: The ‘describe’ method is not part of Angular itself; it's from the Jasmine test framework, which is commonly used for writing tests in Angular applications. The ‘describe’ function is used to group together a set of related tests. It serves as a way to gather tests or specs within a suite which can be about a specific method, component, or module.
Here's how you might use it in a real-world scenario:
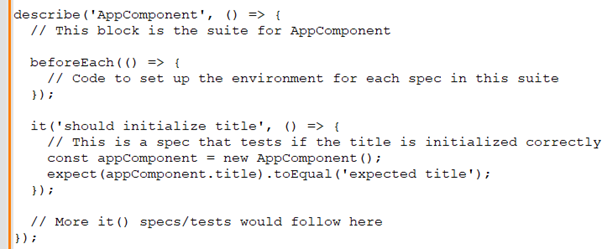
Question: What is beforeEach method?
Answer: The ‘beforeEach’ method in Angular is primarily used within the testing environment, specifically with the Jasmine testing framework. It's a function that is called before each test spec (a single ‘it’ block) is executed. This is incredibly useful for setting up the conditions that should apply to every test, ensuring a consistent starting point for each one. This helps in reducing code repetition and makes the test suite more maintainable.
For instance, if you have multiple tests that need a component to be instantiated before they run, you can put that instantiation code in a ‘beforeEach’ block so that it runs before each test, like so:
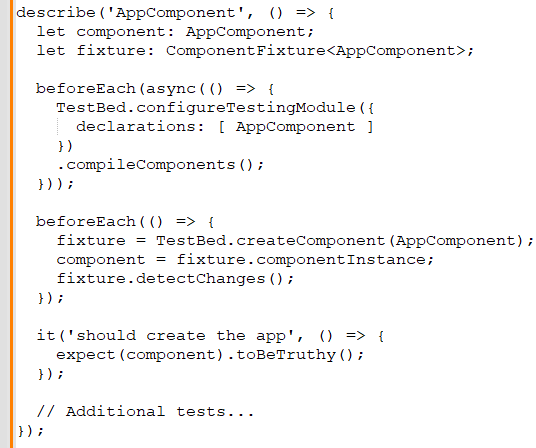
Question: How do you perform test for pipe?
Answer: When testing a pipe in Angular, you typically want to focus on its transformation logic. Let's say we have a ‘capitalizePipe’ that capitalizes the first letter of each word in a string. Here's how you might approach testing it:
- Create a testing module that includes the pipe you're testing. - Instantiate the pipe in your test spec.- Call the ‘transform’ method of the pipe with various inputs to verify that the output is as expected.
Here's an example of how this might look in code:
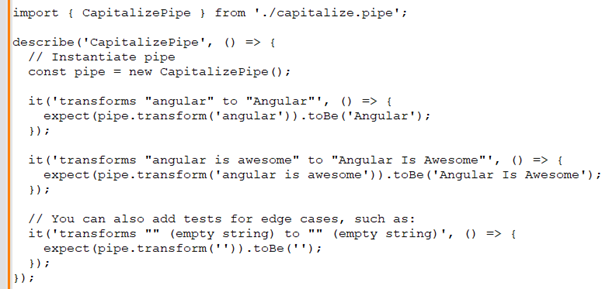
Question: How do you perform test for Services?
Answer: In Angular, services are typically tested using unit tests. To perform tests on a service, you would use the Angular testing utilities alongside a testing framework like Jasmine. Here's how you approach it:
- Set up the Testing Module: You first need to configure the ‘TestBed’, which is the primary API for writing unit tests in Angular. You'll configure it to know about the service you're testing.
- Inject the Service: Using the TestBed, you inject the service into your test to make it available.
- Write Test Cases: You then write your test cases, using ‘it()’ blocks, where you call methods on your service and use ‘expect()’ to assert expected outcomes.
- Mock Dependencies: If your service has dependencies, you'll typically mock these using spies or mock classes.
- Test Asynchronous Operations: If the service returns ‘Observable’s or uses promises, you'll handle them using ‘async’ and ‘await’, or ‘fakeAsync’ and ‘tick()’.
Here's a simple example of what a test for a hypothetical ‘AuthService’ that has a ‘login()’ method might look like:
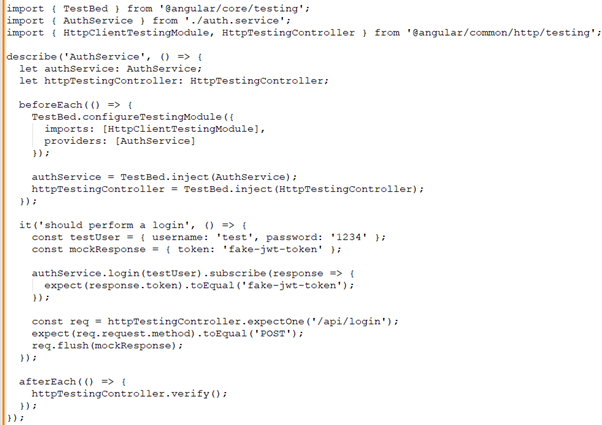
ReadioBook.com