Chapter 2: Python Mock Interview Second Round.
Introduction: Welcome to this pivotal chapter in our audiobook, where we dive into the stimulating world of technical interviews for a seasoned Python Developer. As we turn the page to this section, we immerse ourselves in the narrative of Sonal, a proficient developer, as she navigates the rigorous layers of a job selection process.
The chapter meticulously unfolds across multiple rounds of interviews, showcasing the depth of inquiry and expertise required for a prestigious role within a large enterprise.
Listeners will be privy to a series of thoughtfully crafted questions and responses that encapsulate the essence of a real-life interview scenario.
From foundational queries to complex problem-solving discussions, each segment is designed to not only evaluate technical acumen but also to reveal Sonal's analytical prowess and adaptability to emerging technologies.As the chapter progresses, the stakes heighten with Sonal's exemplary performance in the initial rounds, leading to an anticipated final discussion with a Senior Manager.
This chapter is not just an examination of Sonal's journey but also serves as a comprehensive guide for aspiring Python developers who seek to understand what it takes to excel in such a dynamic and demanding field.Engage with the narrative as it provides invaluable insights into the technicalities of Python development, the strategic mindset required for effective problem-solving, and the interpersonal skills necessary to thrive in a collaborative work environment.
This chapter is a testament to the rigorous standards and the exhilarating challenges that define the path to becoming a successful Python Developer in today's competitive job market.
John: Sonal, can you describe how you would implement a microservices architecture in Python?
Sonal: Certainly, John. In Python, a microservices architecture can be implemented using frameworks like Flask or FastAPI, which allow for the creation of lightweight services that communicate via RESTful APIs or asynchronous message brokers like RabbitMQ or Kafka. Each microservice would be developed around a specific business capability, run in its own process, and communicate with lightweight mechanisms, often an HTTP resource API.
John: That's a solid foundation. Now, can you explain the role of a message broker like Kafka in a Python application?
Sonal: A message broker like Kafka acts as an intermediary for message queues and topics. In a Python application, it can be used for building event-driven architectures, enabling services to publish and subscribe to streams of records or events. Kafka helps in decoupling the services, improving scalability, and ensuring reliable data transfer.
John: Great. How would you optimize a Django application's performance?
Sonal: To optimize a Django application, I would start with database optimization, using indexing and query optimization. I would also use Django's caching mechanisms to reduce database hits. Additionally, I would employ middleware for compression, use a CDN for static files, and profile the application to identify and fix bottlenecks.
John: Impressive. What strategies do you employ for debugging a complex codebase?
Sonal: When debugging a complex codebase, I systematically isolate the issue using logging and Python's debugging tools like pdb. I also write unit tests to narrow down the problem area. Code reviews and pair programming can be extremely helpful in such situations to gain different perspectives.
John: Can you explain how you would use Docker in a development environment?
Sonal: Docker can be used to containerize an application and its environment. For development, it ensures that all team members work with the same environment, reducing the "it works on my machine" problem. It simplifies dependency management and can easily spin up the necessary services like databases or message brokers that the application depends on.
John: What is your approach to writing unit tests in Python?
Sonal: My approach to writing unit tests in Python is to use the ‘unittest’ framework. I ensure that tests are isolated, repeatable, and cover both happy and edge case scenarios. I also employ Test-Driven Development (TDD) when possible, writing tests before the actual code.
John: Lastly, can you discuss how you ensure the security of REST APIs?
Sonal: Ensuring the security of REST APIs involves multiple strategies. I use HTTPS to encrypt data in transit, employ token-based authentication, and implement proper error handling to avoid leaking information. It's also crucial to validate and sanitize all input to prevent injections and use API rate limiting to defend against DDoS attacks.
John: In your experience, how important is code readability, and how do you achieve it in Python?
Sonal: Code readability is paramount in Python, as it directly impacts maintainability and collaboration. I adhere to PEP 8 style guidelines, use meaningful variable and function names, and write concise comments and docstrings where necessary. I also structure my code with proper indentation and spacing, making it easily navigable and understandable by others.
John: Can you walk me through the process of profiling a Python application for performance issues?
Sonal: Profiling involves using tools like ‘cProfile’ to record various statistics about the function calls made in a program. I start by running the profiler on the application to identify slow spots, looking at metrics like call count, cumulative time, and per-call time. Based on the profile report, I focus on optimizing the most time-consuming parts of the code, which often yields the most significant performance improvements.
John: How do you approach error handling and logging in a large-scale Python application?
Sonal: For error handling, I use Python's try-except blocks to catch exceptions and handle them gracefully. I ensure that the application fails safely without exposing sensitive information. For logging, I use Python's built-in logging module, configuring different log levels and handlers to record messages that are informative and useful for troubleshooting.
John: Can you describe the concept of "Don't Repeat Yourself" (DRY) and how you apply it in Python programming?
Sonal: The DRY principle is about reducing repetition of software patterns. In Python, I follow this principle by modularizing code, creating reusable functions, and utilizing inheritance and composition where appropriate. This not only minimizes redundancy but also facilitates changes, as a single modification can propagate throughout the codebase.
John: How would you implement continuous integration/continuous deployment (CI/CD) in a Python project?
Sonal: Implementing CI/CD in a Python project typically involves using tools like Jenkins, GitLab CI, or GitHub Actions. I would set up automated pipelines that run tests, lint code, and deploy applications to staging or production environments. These pipelines would be triggered on every commit or pull request to ensure that the codebase remains stable and deployable at all times.
John: Explain how you've used multithreading or multiprocessing in Python, and what are the challenges associated with it?
Sonal: In Python, I've used the ‘threading’ module for I/O-bound tasks to run multiple operations concurrently. For CPU-bound tasks, I've used the ‘multiprocessing’ module to leverage multiple CPU cores. The main challenge is ensuring thread-safe operations to prevent race conditions, which I handle through synchronization primitives like locks.
With multiprocessing, the challenge is often related to the overhead of starting processes and sharing data between them, which I manage using IPC mechanisms provided by Python.
John: Describe an experience where you had to refactor legacy code. What approach did you take?
Sonal: I once worked on a legacy system where the codebase was not following modern best practices. My approach was systematic: I started by understanding the existing functionality and then incrementally refactored the code module by module, ensuring not to break any features. I wrote tests before refactoring to ensure that functionality remained consistent and utilized principles like encapsulation and modular design to improve the codebase's maintainability.
John: What are some of the advantages of using asynchronous programming in Python, and how have you implemented it?
Sonal: Asynchronous programming is beneficial for I/O-bound and high-latency operations as it improves the efficiency and responsiveness of applications. In Python, I've implemented asynchronous programming using the ‘asyncio’ library and the ‘async/await’ syntax. This was particularly useful in a web scraping project where I could concurrently make multiple HTTP requests and handle responses as they arrived, significantly speeding up the process.
John: How do you approach dependency management in Python projects?
Sonal: For dependency management, I use virtual environments, typically with ‘venv’ to isolate project-specific dependencies. I also use ‘pip’ for package installation and ‘requirements.txt’ or ‘Pipfile’ for specifying project dependencies. For larger projects, I might use a tool like ‘pip-tools’ or ‘Poetry’ to manage dependencies more robustly, ensuring consistent development, testing, and production environments.
John: In your view, what are some best practices for API versioning?
Sonal: API versioning is crucial for maintaining backward compatibility and allowing clients to transition at their own pace. I usually follow the URL versioning approach, where the API version is part of the URL path. Another method I've used is Accept header versioning, where the version is specified in the HTTP headers.
It's also important to clearly document the changes in each version and provide deprecation notices well in advance.
John: When would you choose NoSQL over a traditional SQL database for a project?
Sonal: I would choose a NoSQL database when the project requires high throughput and scalability, especially for big data applications or when the data is unstructured or semi-structured. NoSQL databases offer flexible schemas which are useful when the data model is evolving rapidly.
John: What strategies would you use to ensure that your Python code is secure?
Sonal: To secure my Python code, I would use static code analysis tools to detect vulnerabilities, follow the principle of least privilege when accessing resources, sanitize all inputs to prevent injection attacks, and keep dependencies up to date. Additionally, I would employ proper exception handling to avoid leaking sensitive information.
John: How do you ensure that your Python code adheres to both functional and non-functional requirements?
Sonal: I ensure adherence to functional requirements by writing clear and concise code that fulfills the specified business logic and by thorough testing. For non-functional requirements, such as performance and maintainability, I follow best coding practices, use design patterns appropriately, and profile the code to optimize it.
John: What is your experience with using cloud services in Python applications?
Sonal: I have experience deploying Python applications on cloud platforms like AWS, using services like EC2 for compute resources, S3 for storage, and RDS for managed database services. I've also used serverless architectures with AWS Lambda and API Gateway for building microservices.
John: Describe a time when you had to optimize a slow-running Python script. What approach did you take?
Sonal: I encountered a slow-running script due to inefficient data processing. I profiled the script using cProfile to identify the bottlenecks, optimized the algorithm to reduce time complexity, and replaced some of the data structures with more efficient ones, like using sets instead of lists for membership tests.
John: Can you explain the difference between class-based views and function-based views in Django?
Sonal: Class-based views in Django are more suited for handling complex workflows as they provide a more structured approach and reuse common functionality through inheritance. Function-based views are simpler and more explicit, which can be advantageous for straightforward use cases where you just need to process a request and return a response.
John: How would you handle schema migrations in a production database?
Sonal: Schema migrations in a production database should be handled carefully to avoid downtime. I use Django's migration framework to generate and apply migrations. For critical changes, I would perform dry runs in a staging environment, ensure backups are in place, and choose low-traffic periods for execution.
John: Explain how you have implemented RESTful services in a legacy system.
Sonal: For integrating RESTful services into a legacy system, I started by wrapping the existing functionality with a RESTful API layer. This was done using Flask. I ensured the new layer was stateless and used HTTP methods meaningfully to represent CRUD operations, following REST principles.
John: How do you manage state in a stateless protocol like HTTP?
Sonal: In stateless protocols like HTTP, the server does not retain state between requests. To manage state, I use tokens or cookies for session management, and pass state information through request headers, query string parameters, or in the request body.
John: Discuss your approach to handling real-time data processing in Python.
Sonal: For real-time data processing, I use asynchronous programming with asyncio or frameworks like Tornado. In addition, I leverage message queues like RabbitMQ for decoupling data producers and consumers, and use WebSocket for full-duplex communication between the server and clients.
John: How do you stay updated with the latest Python developments and best practices?
Sonal: I regularly follow Python-related forums, attend webinars, and contribute to open-source projects. I also read PEPs (Python Enhancement Proposals) to stay informed about the latest features in Python and follow the writings and talks of core Python developers.
John: Describe a scenario where you had to use Python decorators. What was the use case?
Sonal: I used decorators to implement authentication and authorization in a web API. The decorators were applied to route handlers to check if the user was logged in and had the correct permissions before allowing access to certain endpoints.
John: Can you talk about a challenging problem you solved using Python's concurrency tools?
Sonal: I once worked on an application that needed to process large files concurrently. I used Python's concurrent.futures module with thread pools to speed up the processing time significantly while avoiding the Global Interpreter Lock (GIL) by doing I/O-bound operations.
John: How do you document your Python code for better maintainability?
Sonal: I use docstrings for modules, classes, functions, and methods. I also follow the Sphinx syntax so that documentation can be automatically generated. Additionally, I write README files and in-line comments where necessary for complex logic.
John: Explain how you would use Python in a data analysis project.
Sonal: In a data analysis project, I would use libraries like pandas for data manipulation, NumPy for numerical computation, and Matplotlib or Seaborn for data visualization. I would also use Jupyter Notebooks for iterative exploration and sharing results.
John: What are some of the Python libraries you've used for web scraping, and how do you handle anti-scraping mechanisms?
Sonal: I've used Beautiful Soup and Scrapy for web scraping. To handle anti-scraping mechanisms, I rotate user agents, use proxies, respect robots.txt, and implement delay between requests. If necessary, I also use Selenium to render JavaScript-heavy pages.
John: How do you approach internationalization (i18n) in Python applications?
Sonal: For internationalization, I use the gettext module in Python to mark translatable strings and then use tools like GNU gettext to manage the translations. Django also has a robust i18n system that I use for web applications.
John: Discuss your experience with Python's metaprogramming features.
Sonal: I've used metaclasses to customize class creation in Python for scenarios like singleton patterns, registering subclasses, and adding new methods dynamically. I also use decorators and the functools.wraps decorator to create function decorators that don't alter the original function's metadata.
John: How have you used Python for system administration tasks?
Sonal: I have automated repetitive system administration tasks with Python by writing scripts that interact with the operating system using the os and subprocess modules. Tasks include file system operations, managing processes, and configuring network settings.
John: What's your process for optimizing the memory usage of a Python application?
Sonal: To optimize memory usage, I first profile the application to identify memory hotspots using tools like memory_profiler. I then look into optimizing data structures, using generators instead of lists where appropriate, and cleaning up circular references. Additionally, I might use __slots__ to reduce the size of objects if a large number of instances are created.
John: Sonal, how do you ensure code quality and maintainability when working in a team?
Sonal: I advocate for code reviews, adherence to a style guide, and comprehensive testing. Using version control systems like Git also helps in managing changes and collaboration. Regular refactoring sessions and documentation also contribute to maintaining code quality.
John: Can you explain the role of the Global Interpreter Lock (GIL) in Python and how it affects multithreaded programs?
Sonal: The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once. This means that threads are not truly concurrent in a multi-threaded application, which can be a bottleneck for CPU-bound programs. To work around this, I use multiprocessing or a multi-process architecture.
John: Describe your experience with Python's virtual environments. Why are they important?
Sonal: Virtual environments in Python are essential for dependency management. They allow you to create isolated environments with specific versions of libraries, avoiding version conflicts between projects. I've extensively used venv and virtualenv to manage project-specific dependencies.
John: In what situations would you recommend using a Python generator?
Sonal: I recommend using generators when working with large data sets that don't fit into memory, or when you want to lazily generate values on the fly. This is more memory-efficient than building and storing the entire collection in memory.
John: How have you utilized Python's exception handling to improve application reliability?
Sonal: I use try-except blocks to catch and handle exceptions gracefully, ensuring the application can recover from unexpected states. I also use finally blocks or context managers to clean up resources like files or network connections, even when an error occurs.
John: What is your approach to resolving merge conflicts in a version control system like Git?
Sonal: When resolving merge conflicts, I first analyze the changes from both sides to understand the context. I then manually merge the changes, ensuring that the code remains functional and consistent with the intended behavior. Communication with team members is also key to avoid overlapping work.
John: How would you monitor and improve the performance of a live Python web application?
Sonal: I would use monitoring tools like New Relic or Datadog to track performance metrics. For improvements, I'd look into optimizing database queries, using caching, load balancing, and code profiling to identify and optimize slow code paths.
John: Share an instance where you had to use design patterns in Python. Which pattern did you use and why?
Sonal: I've used the Factory pattern to provide a way to instantiate objects without specifying the exact class of object that will be created, which is helpful when the system needs to be flexible and extendable. The Singleton pattern is another one I've used to ensure a class has only one instance.
John: What's your approach to testing and validating Python data analysis scripts?
Sonal: For data analysis scripts, I write tests to validate the data processing logic, ensuring the correctness of the scripts. I also perform exploratory data analysis to manually inspect the data and validate results. Using assertions within the script helps catch data anomalies.
John: How do you stay up to date with the latest trends and technologies in software development?
Sonal: I follow industry blogs, participate in tech meetups, contribute to open-source projects, and take online courses to stay up to date. I also experiment with new technologies on personal projects.
John: Sonal, you've handled these technical questions with depth and clarity, indicating a strong command of Python and software development practices. Based on your performance, we'd like to advance you to the next round, where you'll meet with our Senior Manager. This will be the final technical discussion before potential team placements.
Keep up the excellent work, and we look forward to seeing how you'll bring your skills to more complex challenges in the next interview. Sonal: Thank you John!
Introduction: Welcome to this pivotal chapter in our audiobook, where we dive into the stimulating world of technical interviews for a seasoned Python Developer. As we turn the page to this section, we immerse ourselves in the narrative of Sonal, a proficient developer, as she navigates the rigorous layers of a job selection process.
The chapter meticulously unfolds across multiple rounds of interviews, showcasing the depth of inquiry and expertise required for a prestigious role within a large enterprise.
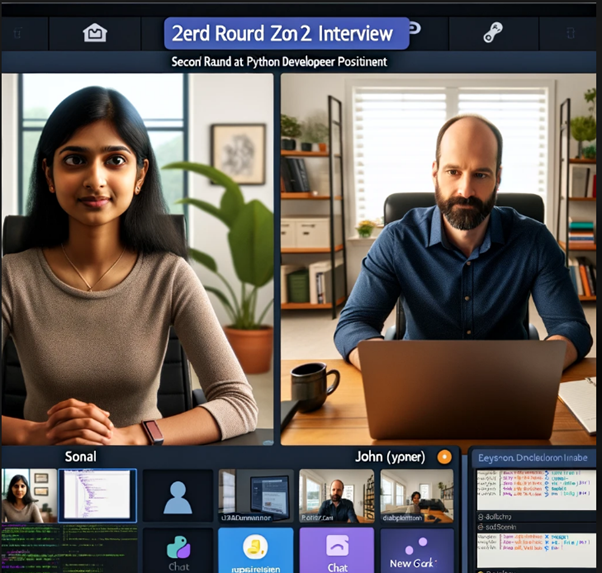
John: Sonal, can you describe how you would implement a microservices architecture in Python?
Sonal: Certainly, John. In Python, a microservices architecture can be implemented using frameworks like Flask or FastAPI, which allow for the creation of lightweight services that communicate via RESTful APIs or asynchronous message brokers like RabbitMQ or Kafka. Each microservice would be developed around a specific business capability, run in its own process, and communicate with lightweight mechanisms, often an HTTP resource API.
John: That's a solid foundation. Now, can you explain the role of a message broker like Kafka in a Python application?
Sonal: A message broker like Kafka acts as an intermediary for message queues and topics. In a Python application, it can be used for building event-driven architectures, enabling services to publish and subscribe to streams of records or events. Kafka helps in decoupling the services, improving scalability, and ensuring reliable data transfer.
John: Great. How would you optimize a Django application's performance?
Sonal: To optimize a Django application, I would start with database optimization, using indexing and query optimization. I would also use Django's caching mechanisms to reduce database hits. Additionally, I would employ middleware for compression, use a CDN for static files, and profile the application to identify and fix bottlenecks.
John: Impressive. What strategies do you employ for debugging a complex codebase?
Sonal: When debugging a complex codebase, I systematically isolate the issue using logging and Python's debugging tools like pdb. I also write unit tests to narrow down the problem area. Code reviews and pair programming can be extremely helpful in such situations to gain different perspectives.
John: Can you explain how you would use Docker in a development environment?
Sonal: Docker can be used to containerize an application and its environment. For development, it ensures that all team members work with the same environment, reducing the "it works on my machine" problem. It simplifies dependency management and can easily spin up the necessary services like databases or message brokers that the application depends on.
John: What is your approach to writing unit tests in Python?
Sonal: My approach to writing unit tests in Python is to use the ‘unittest’ framework. I ensure that tests are isolated, repeatable, and cover both happy and edge case scenarios. I also employ Test-Driven Development (TDD) when possible, writing tests before the actual code.
John: Lastly, can you discuss how you ensure the security of REST APIs?
Sonal: Ensuring the security of REST APIs involves multiple strategies. I use HTTPS to encrypt data in transit, employ token-based authentication, and implement proper error handling to avoid leaking information. It's also crucial to validate and sanitize all input to prevent injections and use API rate limiting to defend against DDoS attacks.
John: In your experience, how important is code readability, and how do you achieve it in Python?
Sonal: Code readability is paramount in Python, as it directly impacts maintainability and collaboration. I adhere to PEP 8 style guidelines, use meaningful variable and function names, and write concise comments and docstrings where necessary. I also structure my code with proper indentation and spacing, making it easily navigable and understandable by others.
John: Can you walk me through the process of profiling a Python application for performance issues?
Sonal: Profiling involves using tools like ‘cProfile’ to record various statistics about the function calls made in a program. I start by running the profiler on the application to identify slow spots, looking at metrics like call count, cumulative time, and per-call time. Based on the profile report, I focus on optimizing the most time-consuming parts of the code, which often yields the most significant performance improvements.
John: How do you approach error handling and logging in a large-scale Python application?
Sonal: For error handling, I use Python's try-except blocks to catch exceptions and handle them gracefully. I ensure that the application fails safely without exposing sensitive information. For logging, I use Python's built-in logging module, configuring different log levels and handlers to record messages that are informative and useful for troubleshooting.
John: Can you describe the concept of "Don't Repeat Yourself" (DRY) and how you apply it in Python programming?
Sonal: The DRY principle is about reducing repetition of software patterns. In Python, I follow this principle by modularizing code, creating reusable functions, and utilizing inheritance and composition where appropriate. This not only minimizes redundancy but also facilitates changes, as a single modification can propagate throughout the codebase.
John: How would you implement continuous integration/continuous deployment (CI/CD) in a Python project?
Sonal: Implementing CI/CD in a Python project typically involves using tools like Jenkins, GitLab CI, or GitHub Actions. I would set up automated pipelines that run tests, lint code, and deploy applications to staging or production environments. These pipelines would be triggered on every commit or pull request to ensure that the codebase remains stable and deployable at all times.
John: Explain how you've used multithreading or multiprocessing in Python, and what are the challenges associated with it?
Sonal: In Python, I've used the ‘threading’ module for I/O-bound tasks to run multiple operations concurrently. For CPU-bound tasks, I've used the ‘multiprocessing’ module to leverage multiple CPU cores. The main challenge is ensuring thread-safe operations to prevent race conditions, which I handle through synchronization primitives like locks.
With multiprocessing, the challenge is often related to the overhead of starting processes and sharing data between them, which I manage using IPC mechanisms provided by Python.
John: Describe an experience where you had to refactor legacy code. What approach did you take?
Sonal: I once worked on a legacy system where the codebase was not following modern best practices. My approach was systematic: I started by understanding the existing functionality and then incrementally refactored the code module by module, ensuring not to break any features. I wrote tests before refactoring to ensure that functionality remained consistent and utilized principles like encapsulation and modular design to improve the codebase's maintainability.
John: What are some of the advantages of using asynchronous programming in Python, and how have you implemented it?
Sonal: Asynchronous programming is beneficial for I/O-bound and high-latency operations as it improves the efficiency and responsiveness of applications. In Python, I've implemented asynchronous programming using the ‘asyncio’ library and the ‘async/await’ syntax. This was particularly useful in a web scraping project where I could concurrently make multiple HTTP requests and handle responses as they arrived, significantly speeding up the process.
John: How do you approach dependency management in Python projects?
Sonal: For dependency management, I use virtual environments, typically with ‘venv’ to isolate project-specific dependencies. I also use ‘pip’ for package installation and ‘requirements.txt’ or ‘Pipfile’ for specifying project dependencies. For larger projects, I might use a tool like ‘pip-tools’ or ‘Poetry’ to manage dependencies more robustly, ensuring consistent development, testing, and production environments.
John: In your view, what are some best practices for API versioning?
Sonal: API versioning is crucial for maintaining backward compatibility and allowing clients to transition at their own pace. I usually follow the URL versioning approach, where the API version is part of the URL path. Another method I've used is Accept header versioning, where the version is specified in the HTTP headers.
It's also important to clearly document the changes in each version and provide deprecation notices well in advance.
John: When would you choose NoSQL over a traditional SQL database for a project?
Sonal: I would choose a NoSQL database when the project requires high throughput and scalability, especially for big data applications or when the data is unstructured or semi-structured. NoSQL databases offer flexible schemas which are useful when the data model is evolving rapidly.
John: What strategies would you use to ensure that your Python code is secure?
Sonal: To secure my Python code, I would use static code analysis tools to detect vulnerabilities, follow the principle of least privilege when accessing resources, sanitize all inputs to prevent injection attacks, and keep dependencies up to date. Additionally, I would employ proper exception handling to avoid leaking sensitive information.
John: How do you ensure that your Python code adheres to both functional and non-functional requirements?
Sonal: I ensure adherence to functional requirements by writing clear and concise code that fulfills the specified business logic and by thorough testing. For non-functional requirements, such as performance and maintainability, I follow best coding practices, use design patterns appropriately, and profile the code to optimize it.
John: What is your experience with using cloud services in Python applications?
Sonal: I have experience deploying Python applications on cloud platforms like AWS, using services like EC2 for compute resources, S3 for storage, and RDS for managed database services. I've also used serverless architectures with AWS Lambda and API Gateway for building microservices.
John: Describe a time when you had to optimize a slow-running Python script. What approach did you take?
Sonal: I encountered a slow-running script due to inefficient data processing. I profiled the script using cProfile to identify the bottlenecks, optimized the algorithm to reduce time complexity, and replaced some of the data structures with more efficient ones, like using sets instead of lists for membership tests.
John: Can you explain the difference between class-based views and function-based views in Django?
Sonal: Class-based views in Django are more suited for handling complex workflows as they provide a more structured approach and reuse common functionality through inheritance. Function-based views are simpler and more explicit, which can be advantageous for straightforward use cases where you just need to process a request and return a response.
John: How would you handle schema migrations in a production database?
Sonal: Schema migrations in a production database should be handled carefully to avoid downtime. I use Django's migration framework to generate and apply migrations. For critical changes, I would perform dry runs in a staging environment, ensure backups are in place, and choose low-traffic periods for execution.
John: Explain how you have implemented RESTful services in a legacy system.
Sonal: For integrating RESTful services into a legacy system, I started by wrapping the existing functionality with a RESTful API layer. This was done using Flask. I ensured the new layer was stateless and used HTTP methods meaningfully to represent CRUD operations, following REST principles.
John: How do you manage state in a stateless protocol like HTTP?
Sonal: In stateless protocols like HTTP, the server does not retain state between requests. To manage state, I use tokens or cookies for session management, and pass state information through request headers, query string parameters, or in the request body.
John: Discuss your approach to handling real-time data processing in Python.
Sonal: For real-time data processing, I use asynchronous programming with asyncio or frameworks like Tornado. In addition, I leverage message queues like RabbitMQ for decoupling data producers and consumers, and use WebSocket for full-duplex communication between the server and clients.
John: How do you stay updated with the latest Python developments and best practices?
Sonal: I regularly follow Python-related forums, attend webinars, and contribute to open-source projects. I also read PEPs (Python Enhancement Proposals) to stay informed about the latest features in Python and follow the writings and talks of core Python developers.
John: Describe a scenario where you had to use Python decorators. What was the use case?
Sonal: I used decorators to implement authentication and authorization in a web API. The decorators were applied to route handlers to check if the user was logged in and had the correct permissions before allowing access to certain endpoints.
John: Can you talk about a challenging problem you solved using Python's concurrency tools?
Sonal: I once worked on an application that needed to process large files concurrently. I used Python's concurrent.futures module with thread pools to speed up the processing time significantly while avoiding the Global Interpreter Lock (GIL) by doing I/O-bound operations.
John: How do you document your Python code for better maintainability?
Sonal: I use docstrings for modules, classes, functions, and methods. I also follow the Sphinx syntax so that documentation can be automatically generated. Additionally, I write README files and in-line comments where necessary for complex logic.
John: Explain how you would use Python in a data analysis project.
Sonal: In a data analysis project, I would use libraries like pandas for data manipulation, NumPy for numerical computation, and Matplotlib or Seaborn for data visualization. I would also use Jupyter Notebooks for iterative exploration and sharing results.
John: What are some of the Python libraries you've used for web scraping, and how do you handle anti-scraping mechanisms?
Sonal: I've used Beautiful Soup and Scrapy for web scraping. To handle anti-scraping mechanisms, I rotate user agents, use proxies, respect robots.txt, and implement delay between requests. If necessary, I also use Selenium to render JavaScript-heavy pages.
John: How do you approach internationalization (i18n) in Python applications?
Sonal: For internationalization, I use the gettext module in Python to mark translatable strings and then use tools like GNU gettext to manage the translations. Django also has a robust i18n system that I use for web applications.
John: Discuss your experience with Python's metaprogramming features.
Sonal: I've used metaclasses to customize class creation in Python for scenarios like singleton patterns, registering subclasses, and adding new methods dynamically. I also use decorators and the functools.wraps decorator to create function decorators that don't alter the original function's metadata.
John: How have you used Python for system administration tasks?
Sonal: I have automated repetitive system administration tasks with Python by writing scripts that interact with the operating system using the os and subprocess modules. Tasks include file system operations, managing processes, and configuring network settings.
John: What's your process for optimizing the memory usage of a Python application?
Sonal: To optimize memory usage, I first profile the application to identify memory hotspots using tools like memory_profiler. I then look into optimizing data structures, using generators instead of lists where appropriate, and cleaning up circular references. Additionally, I might use __slots__ to reduce the size of objects if a large number of instances are created.
John: Sonal, how do you ensure code quality and maintainability when working in a team?
Sonal: I advocate for code reviews, adherence to a style guide, and comprehensive testing. Using version control systems like Git also helps in managing changes and collaboration. Regular refactoring sessions and documentation also contribute to maintaining code quality.
John: Can you explain the role of the Global Interpreter Lock (GIL) in Python and how it affects multithreaded programs?
Sonal: The GIL is a mutex that protects access to Python objects, preventing multiple threads from executing Python bytecodes at once. This means that threads are not truly concurrent in a multi-threaded application, which can be a bottleneck for CPU-bound programs. To work around this, I use multiprocessing or a multi-process architecture.
John: Describe your experience with Python's virtual environments. Why are they important?
Sonal: Virtual environments in Python are essential for dependency management. They allow you to create isolated environments with specific versions of libraries, avoiding version conflicts between projects. I've extensively used venv and virtualenv to manage project-specific dependencies.
John: In what situations would you recommend using a Python generator?
Sonal: I recommend using generators when working with large data sets that don't fit into memory, or when you want to lazily generate values on the fly. This is more memory-efficient than building and storing the entire collection in memory.
John: How have you utilized Python's exception handling to improve application reliability?
Sonal: I use try-except blocks to catch and handle exceptions gracefully, ensuring the application can recover from unexpected states. I also use finally blocks or context managers to clean up resources like files or network connections, even when an error occurs.
John: What is your approach to resolving merge conflicts in a version control system like Git?
Sonal: When resolving merge conflicts, I first analyze the changes from both sides to understand the context. I then manually merge the changes, ensuring that the code remains functional and consistent with the intended behavior. Communication with team members is also key to avoid overlapping work.
John: How would you monitor and improve the performance of a live Python web application?
Sonal: I would use monitoring tools like New Relic or Datadog to track performance metrics. For improvements, I'd look into optimizing database queries, using caching, load balancing, and code profiling to identify and optimize slow code paths.
John: Share an instance where you had to use design patterns in Python. Which pattern did you use and why?
Sonal: I've used the Factory pattern to provide a way to instantiate objects without specifying the exact class of object that will be created, which is helpful when the system needs to be flexible and extendable. The Singleton pattern is another one I've used to ensure a class has only one instance.
John: What's your approach to testing and validating Python data analysis scripts?
Sonal: For data analysis scripts, I write tests to validate the data processing logic, ensuring the correctness of the scripts. I also perform exploratory data analysis to manually inspect the data and validate results. Using assertions within the script helps catch data anomalies.
John: How do you stay up to date with the latest trends and technologies in software development?
Sonal: I follow industry blogs, participate in tech meetups, contribute to open-source projects, and take online courses to stay up to date. I also experiment with new technologies on personal projects.
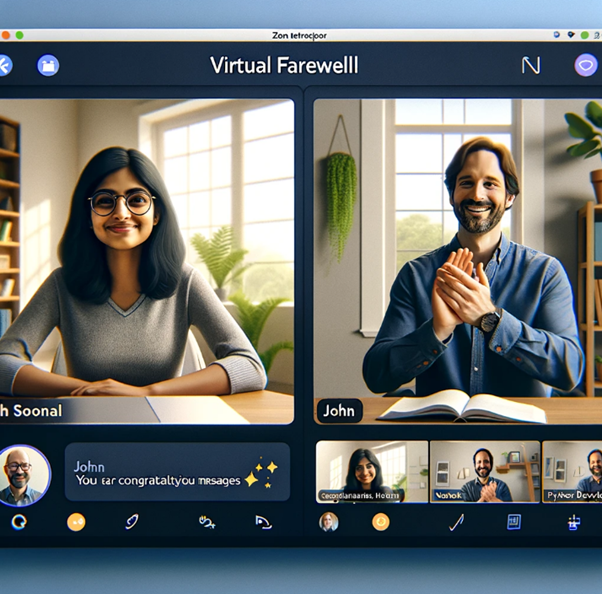
John: Sonal, you've handled these technical questions with depth and clarity, indicating a strong command of Python and software development practices. Based on your performance, we'd like to advance you to the next round, where you'll meet with our Senior Manager. This will be the final technical discussion before potential team placements.
Keep up the excellent work, and we look forward to seeing how you'll bring your skills to more complex challenges in the next interview. Sonal: Thank you John!
ReadioBook.com