Chapter-3: Interview Question for Python Fundamental and OOPs Part 3.
Introduction: In this chapter we are going to cover some more questions on Python Fundamental Concepts and then we will have some questions around Python OOPs concept. So, lets start.
Question: Please explain namespace in Python and how it is being used?
Answer: In Python, a namespace is a mapping where every name, such as a variable, function, class, module, etc., is mapped to an object. Namespaces are used to avoid naming conflicts by ensuring that names in a program are unique and can be used without conflict. Python implements namespaces as dictionaries with 'name as key' mapped to a corresponding 'object as value'.
Namespaces include:
Local Namespace: This includes local names inside a function. The namespace is temporarily created for a function call and gets cleared when the function returns.
Global Namespace: This includes names from various imported packages/modules that are being used in the current project. This namespace is created when the module is included in the script and lasts until the script is executed.
Built-in Namespace: This includes built-in functions and built-in exception names.
In Python, namespaces are created at different moments and have different lifetimes. The scope of a namespace is the region of a Python program where that namespace is directly accessible. The ‘dir()’ function is used to list all the names a namespace contains.
Here’s an example to illustrate how namespaces work:
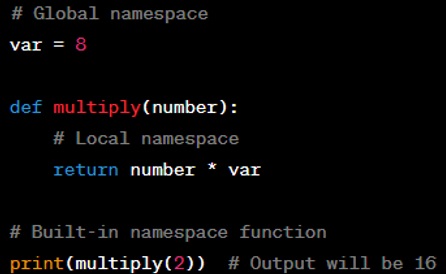
In this example:
- ‘var’ is in the global namespace.- ‘number’ is in the local namespace of the ‘multiply’ function.- ‘print’ and ‘multiply’ are in the built-in namespace.
When you request to access a name, Python searches the namespaces in a specific order: first it checks the local namespace (innermost), then the global namespace (middle), and finally the built-in namespace (outermost), which is why this is often referred to as the LEGB rule (Local, Enclosing, Global, Built-in). Namespaces are an essential feature for avoiding naming conflicts and for facilitating modularity in Python, allowing for the same name to be used for different objects in different contexts.
Question: Why do you use PYTHONPATH environment variable?
Answer: The ‘PYTHONPATH’ environment variable is used to specify additional directories where Python should look for modules and packages. During an interview, if asked why you use the ‘PYTHONPATH’ environment variable, you might answer like this:
I use the ‘PYTHONPATH’ environment variable to add specific directories to Python's search path for modules and packages. This is particularly useful when I am working with Python code that is not installed in the standard location, such as modules developed in-house or when working in a virtual environment. It allows me to run Python code that depends on these modules without having to install them into the global Python environment. Essentially, it helps in managing the module search path dynamically and can be very handy for development and testing purposes.
Question: Why do you want to use help() and dir() function in Python?
Answer: In an interview, you might answer this question as follows:
The ‘help()’ and ‘dir()’ functions are integral to Python programming, especially when dealing with new or unfamiliar objects or modules. Here's why they are important:
The ‘help()’ function is used to understand what something does in Python. It's an in-built function that provides a helpful interface for accessing Python's documentation directly from the interpreter. When you're working with a new module or function, using ‘help()’ can quickly show you its documentation, including the description of the module, the functions it contains, its classes, and more. This can be extremely valuable when you need to get up to speed with unfamiliar code or when you need to remember the details of an infrequently used module.On the other hand, the ‘dir()’ function is used to find out which names a module defines. It returns a sorted list of strings containing the names that the module defines. When a module is large and contains many functions, ‘dir()’ can be particularly useful because it provides a quick way to get an overview of all the attributes that you can work with.Both ‘help()’ and ‘dir()’ are about discovery and understanding. They help to foster a deeper insight into the code, enable better use of the various Python components, and assist in debugging by providing a clearer picture of what’s available and how it’s intended to be used. These functions are especially helpful for beginners who are getting to grips with the language, but even experienced developers find them indispensable when exploring new libraries or refreshing their memory on the details of existing ones.
Question: Please explain what do you mean by Python is an interpreted language?
Answer: When we say that Python is an interpreted language, we mean that Python code is executed by an interpreter rather than being compiled into machine-language instructions that can be executed directly by the computer's CPU. In a compiled language, the source code you write is transformed into machine code by a compiler before it is run on a computer. This machine code is specific to the processor's architecture and is executed directly by the CPU.In contrast, an interpreter for a language like Python directly executes the instructions written in Python code without compiling them into machine code first. When you run a Python program, the interpreter reads the Python code and carries out the actions specified. This means that Python code is not bound to a specific hardware platform, and it is often easier to debug because you can read and interpret the Python code as it runs. However, interpreted languages can be slower than compiled languages because the interpretation needs to happen every time the program is run, rather than just once at the beginning.
Question: When we say argument passed by value and pass by reference, what it means and what are the effects on the same?
Answer: In Python, arguments can be passed to functions either by value or by reference. This concept is crucial in understanding how functions interact with their arguments, and it affects how changes made to arguments inside functions can impact the variables outside the function.
Pass by Value:
- When an argument is passed by value, a copy of the data is made and passed to the function. - Changes made to the argument within the function do not affect the original variable outside the function.- In Python, immutable data types like integers, floats, strings, and tuples are effectively passed by value because their copies cannot be changed.
Pass by Reference:
- When an argument is passed by reference, a function receives a reference to the original data, and no copying occurs. - Changes to the argument within the function can affect the original variable outside the function.- In Python, mutable data types like lists, dictionaries, and sets are passed by reference because the function can directly modify the original data.However, it's essential to note that Python's argument passing is actually more accurately described as "pass by assignment" or "pass by object reference".
This means that function arguments in Python are neither strictly pass by value nor pass by reference. Instead, Python passes the reference to the object (the value itself for immutable objects) to the function. Here's what happens:
- If the object passed is immutable, the function cannot modify the caller's variable, even though it is technically a reference that is passed. - If the object is mutable, the function can modify the caller's variable in place.
To illustrate, consider the following examples:
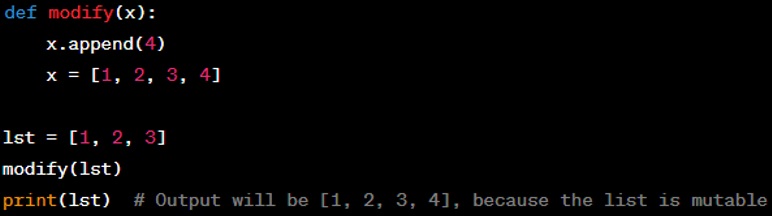
Conversely:
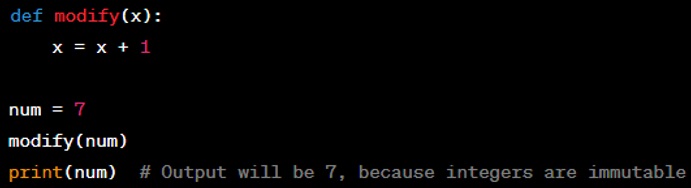
Question: Can you please explain what is iterator?
Answer: In Python, an iterator is an object that enables a programmer to traverse through all the elements of a collection, such as a list or a tuple. Unlike lists or tuples, iterators don’t store their contents in memory; instead, they generate each value one at a time, which means they are more memory efficient when dealing with large datasets. To understand iterators, it's essential to be familiar with the concept of iterables. An iterable is any Python object capable of returning its members one at a time, allowing it to be iterated over in a loop. Common examples of iterables include lists, tuples, and strings.
Here’s a more detailed breakdown:
Iterator Protocol: The iterator protocol in Python involves two methods. The ‘ iter ()’ method, which returns the iterator object itself, is called when the iteration is initialized. The ‘ next ()’ method returns the next value from the sequence. When there are no more items to return, ‘ next ()’ raises a ‘StopIteration’ exception which tells the loop to terminate.
Creating Iterators: You can turn an iterable into an iterator by using the ‘iter()’ function. For example, you could take a list and create an iterator like this: ‘my iterator = iter(my list)’.
Using Iterators: Once you have an iterator, you can use ‘next()’ to get each element one by one. You could also use a for loop, which internally creates an iterator from the iterable and calls ‘next()’ on it until the ‘StopIteration’ exception is raised.
Advantages: Iterators are useful when you have a large dataset and don't want to store the entire thing in memory. They are also a fundamental part of Python's design and are used behind the scenes in loops, comprehensions, and generators.
To sum up, an iterator in Python is a specialized object that provides a way to access elements from a collection one at a time and in a specific order, without requiring the overhead of indexing or the memory use of storing the entire collection at once.
Question: Please explain args and kwargs in Python?
Answer: In Python, ‘*args’ and ‘kwargs’ are special syntax elements used in function definitions. They allow a function to accept an arbitrary number of arguments and keyword arguments respectively.
‘*args’: This is used to pass a non-keyworded, variable-length argument list. The syntax is to use the asterisk (*) before a parameter name when defining a function. Inside the function, ‘args’ is a tuple of the positional arguments that were passed. The arguments are accessed just like an iterable, so you can loop over them, access them by index, etc.
For example, check code below:
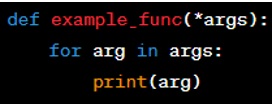
‘kwargs’: This stands for "keyword arguments" and allows you to pass a variable number of keyword arguments to a function. The double asterisk () is used before a parameter name. ‘kwargs’ within the function is a dictionary where keys are the argument names and values are the argument values.
For example, check code below:
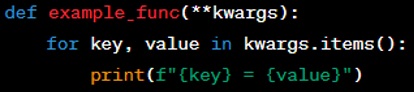
Question: Please explain negative indexes in Python?
Answer: In Python, negative indexing means accessing list elements from the end rather than the beginning. The index ‘-1’ corresponds to the last item in the list, ‘-2’ to the second last, and so on. This is particularly useful when you want to access elements but don't know the length of the list or you want to quickly refer to the elements at the end.
Here's how it works:
- ‘list[-1]’ will give you the last element of the list.- ‘list[-2]’ will give you the second last element of the list.This indexing method is not limited to lists but also works with other sequence types such as strings, tuples, and arrays. It's a feature that provides a convenient way to navigate sequences in Python.
Question: Explain split() and join() functions in Python?
Answer: In Python, ‘split()’ and ‘join()’ are two commonly used string methods for manipulating strings.
The ‘split()’ method is used to break up a string into a list of substrings based on a specified delimiter. If you don't specify a delimiter, it will default to splitting on whitespace.
Here’s how you might explain it in an interview: Imagine you have a sentence and you want to find each individual word. In Python, you could use the ‘split()’ function on the string. For example, ‘'hello world'.split()’ would return ‘['hello', 'world']’. You can also specify a different delimiter, like a comma, to split on that instead.The ‘join()’ method is kind of the inverse of ‘split()’. It takes an iterable like a list of strings and concatenates its elements into a single string, with a specified string acting as the delimiter between elements.
For instance, you might say: If you have a list of words and want to stitch them back into a sentence, the ‘join()’ function is what you'd use. You specify the string to join with, and call ‘join()’ on it, passing the list of strings. So, ‘' '.join(['hello', 'world'])’ would give you the single string ‘'hello world'‘. These methods are very handy for parsing and assembling strings, and they're frequently used in Python for data processing tasks.
Question: Explain how to delete a file in Python?
Answer: In Python, you can delete a file using the ‘os’ module, which provides a way to interface with the underlying operating system. Here's an example of how you can delete a file:
First, you need to import the ‘os’ module:
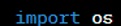
Use the ‘os.remove()’ function, passing the path of the file you want to delete as an argument:

txt'‘ located at ‘'path/to/your/'‘. It's important to note that the file must exist, and your Python script must have the necessary permissions to delete the file, otherwise, an exception will be raised. Always handle exceptions to make your program robust:
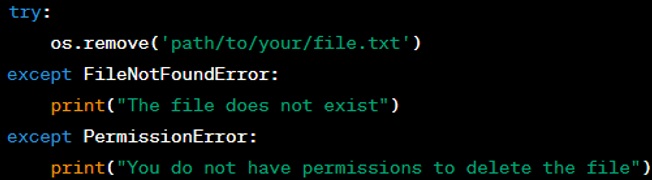
Question: What is a Class in Python?
Answer: In Python, a class is a blueprint for creating objects. Objects have member variables and have behavior associated with them. In python, everything is an object, and classes define the structure and behaviors of these objects. Classes encapsulate data for the object. Here's a simple example of a class in Python:
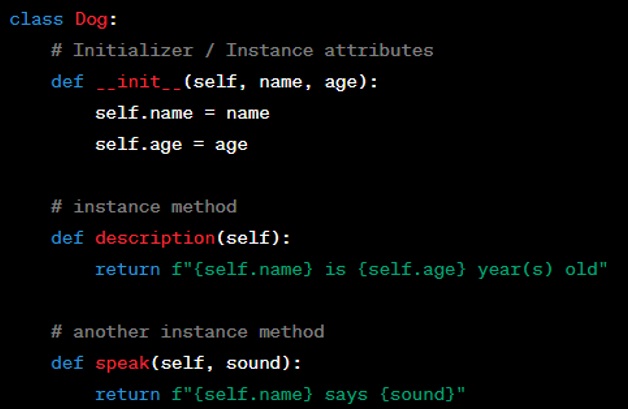
It also has two instance methods: ‘description’ which returns a string containing the dog's name and age, and ‘speak’ which returns a string containing what the dog says. To create an object of this class, you would do something like this:

Question: Please explain inheritance concept in Python with example?
Answer: Inheritance in Python is a concept where a new class is formed using properties and methods from an existing class. The new class is called a derived (or child) class, and the one from which it inherits is called the base (or parent) class. Inheritance enables code reusability and establishes a relationship between the two classes.
Here's an example to illustrate inheritance in Python: Suppose we have a base class called ‘Vehicle’ that represents general attributes and behaviors of a vehicle, like starting the engine or stopping:
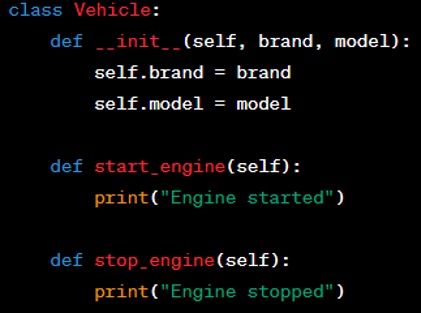
Now, if we want to create a class ‘Car’ that has all the functionalities of ‘Vehicle’ plus some additional features, we can inherit from ‘Vehicle’:
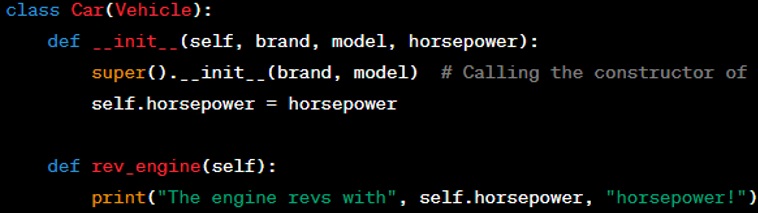
init (brand, model)’ to call the ‘ init ’ method of ‘Vehicle’, thus inheriting its properties. We also add a new method ‘rev engine’ that is specific to ‘Car’. You can use the ‘Car’ class as follows:
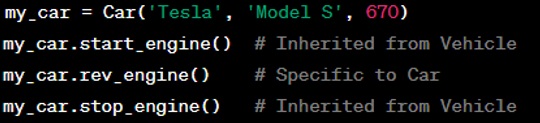
Question: Please explain how do you access parent members from child?
Answer: In Python, when you're dealing with inheritance and you have a child class that inherits from a parent class, you can access the members of the parent class (which includes attributes and methods) directly from an instance of the child class, since they are inherited.
However, if you need to access a member of the parent class that has been overridden in the child class, you use the ‘super()’ function.
‘super()’ returns a temporary object of the superclass that allows you to call its methods. Here's a simple example to illustrate:
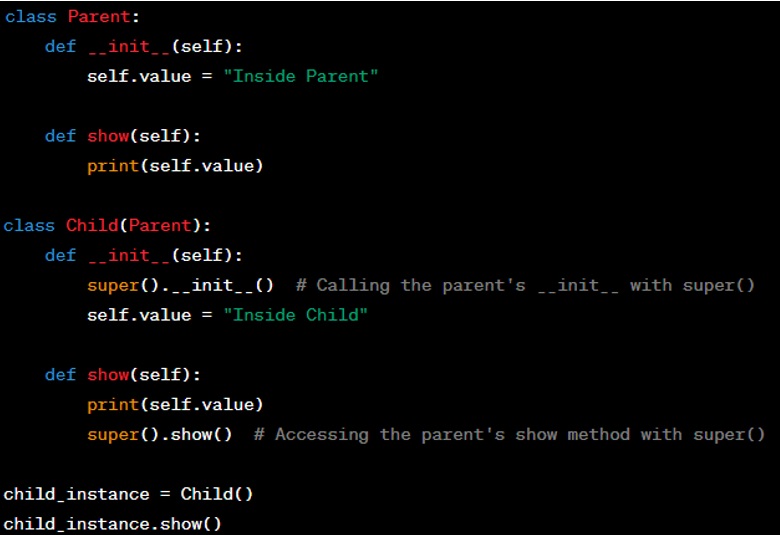
When you run this code, it will output:
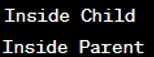
Question: Please explain access modifiers in Python?
Answer: In Python, access modifiers are used to restrict the access to variables and methods within a class. The three types of access modifiers in Python are:
Public Access Modifier: If a member of a class is declared public, it means that it can be freely accessed from inside and outside of the class. In Python, all class variables are public by default.
Protected Access Modifier: Protected members of a class are accessible within the class and are also available to subclasses. In Python, protected members are denoted by prefixing the member name with a single underscore ‘ ’.
Private Access Modifier: Private members are accessible only within the class. This means that they are hidden from outside of the class and cannot be accessed directly. In Python, you denote a private member by prefixing the name with double underscores ‘ ’.
Here's an example to illustrate this:
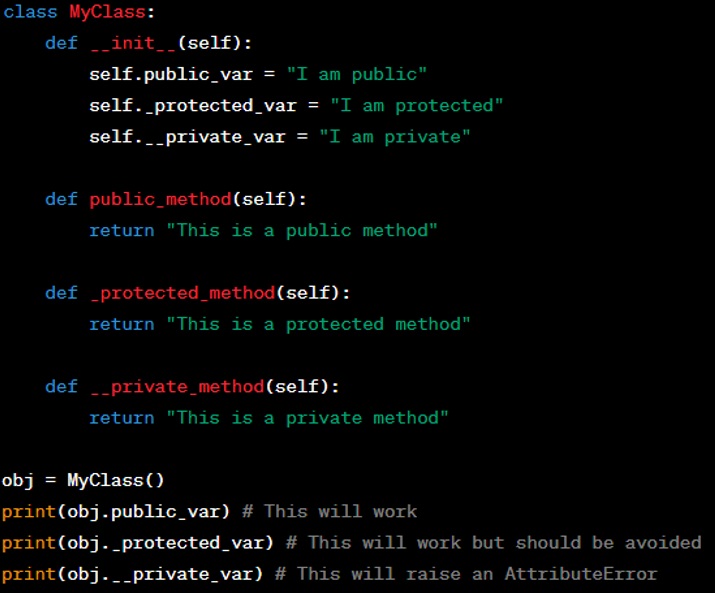
Question: In Python, is it possible to call parent class without its instance creation?
Answer: Yes, in Python, it is possible to call a method from a parent class without creating an instance of the parent. This can be done by directly invoking the method through the class itself, using the ‘super()’ function, or by directly calling the method on the parent class and passing the instance of the child class as the argument.
Here’s how you might use each method:
Using the Parent Class Name: You can call a method of the parent class by directly using the parent class name and passing the instance of the child class to the method.
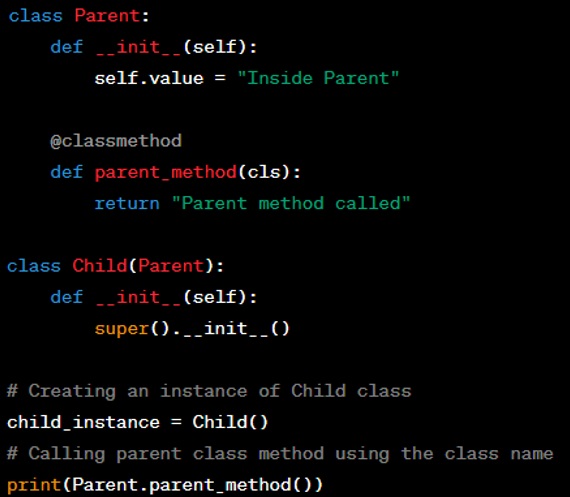
Using the ‘super()’ Function: The ‘super()’ function returns a temporary object of the superclass that allows you to call its methods without needing to instantiate it.
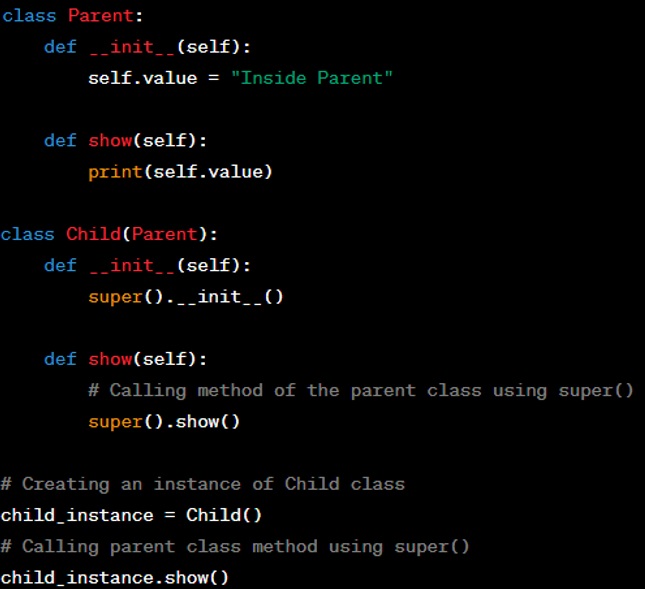
Using ‘super()’ with Class Method: You can use ‘super()’ in a class method as well to call a parent class method that is a class method.
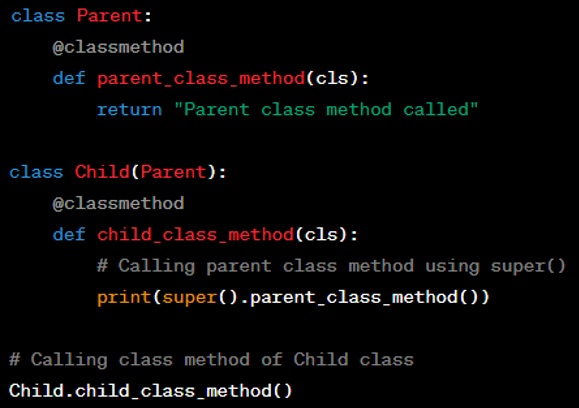
Question: How is an empty class created in python and what its use?
Answer: In Python, an empty class can be created by using the ‘class’ keyword followed by a class name and a pass statement to indicate that no additional attributes or methods are being defined. Here's a simple example of how an empty class, let's name it ‘EmptyClass’, would be defined:
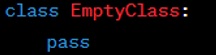
Question: Please explain and differentiate between new and override modifiers.
Answer: In the context of Python programming, the concepts of ‘new’ and ‘override’ modifiers are not native as they are in some other object-oriented languages like C# or Java. Python's approach to method overriding is different, relying on its dynamic nature and the concept of polymorphism.
However, to explain these concepts generally:
- Override refers to the mechanism where a subclass provides a specific implementation of a method that is already defined in its superclass. This is done so that the subclass can provide its version of the method, thus overriding the superclass's version. In Python, method overriding is done simply by defining in the subclass a method with the same name as in the superclass.- New is a modifier found in languages like C#, which indicates that the member is new and not an override of a base class member. The ‘new’ keyword is used to hide a method, property, indexer, or event of the base class, rather than to override it. This concept does not have a direct equivalent in Python, as Python does not have a mechanism for hiding methods in this way.In Python, if you define a method in a subclass with the same name as a method in the superclass, the subclass's method will always override the superclass's method — there's no need to explicitly state this using a modifier like ‘override’. Similarly, there's no concept of ‘new’ because if you want to define a method that doesn't interact with the superclass's method at all, you would simply give it a different name. If you were to emulate ‘new’ behavior in Python, you would just define a method with the same name in the subclass, and it would overshadow the superclass's method, but it would not be considered overriding it, as it would not be involved in polymorphic method calls.
Question: Why is finalize used?
Answer: In Python, there isn't an explicit ‘finalize’ function like in some other languages such as Java; however, Python has a similar concept in the form of a destructor method known as ‘ del ’. This method is called when an object is about to be destroyed, which can happen for various reasons such as when there are no more references to the object or when the program is exiting. The primary use of ‘ del ’, or the finalization process in Python, is to perform any necessary cleanup actions, such as releasing external resources like files or network connections, or explicitly freeing up large resources that the garbage collector might not reclaim immediately. It's important to note that because of Python's garbage collection mechanism, the exact timing of the ‘ del ’ method invocation is not deterministic; thus, relying on it for critical resource cleanup is discouraged. Instead, it's recommended to use context managers and the ‘with’ statement to manage resources, which provide a much more reliable and predictable mechanism for resource management.
Question: What is init method in python?
Answer: The ‘ init ’ method in Python is what's known as a constructor in object-oriented terminology. This method is automatically invoked when a new instance of a class is created. It initializes the object's attributes and serves as the blueprint for setting up the object with its initial state.
Here's what typically happens with an ‘ init ’ method:
- Initialization: It sets up the initial values of instance variables that are necessary for the object to function correctly.
- Object Creation: While the actual object is created before the ‘ init ’ method is called, this method is used to perform any additional setup that the user wants to happen upon object creation.
- Parameter Passing: It allows the class to accept parameters when an object is created, which can then be used to initialize object attributes.
Here's a simple example of a class with an ‘ init ’ method:
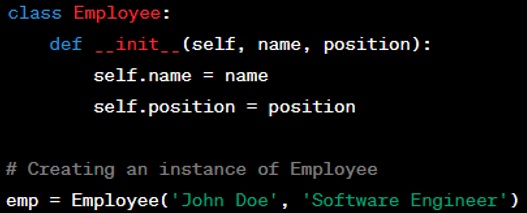
Question: How will you check if a class is a child of another class?
Answer: In Python, you can check if a class is a child of another class (i.e., if a class is a subclass of another class) using the built-in function ‘issubclass()’. The ‘issubclass()’ function takes two arguments. The first argument is the class you want to check, and the second argument is the parent class. It returns ‘True’ if the first class is a subclass of the second class, and ‘False’ otherwise.
Here's how you can use ‘issubclass()’:
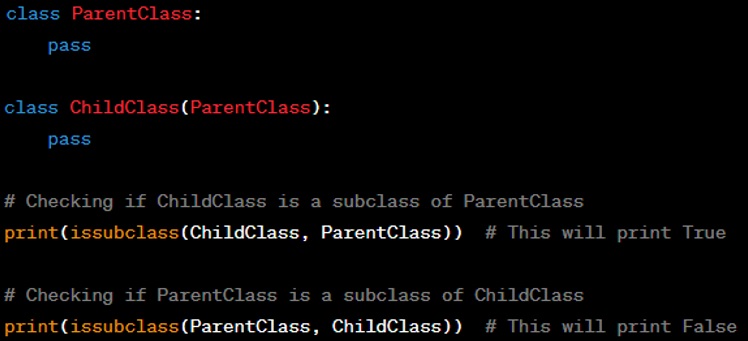
Question: How do you concatenate strings in Python?
Answer: In Python, you can concatenate strings using the ‘+’ operator. For example, if you have two strings, ‘str1 = "Hello"‘ and ‘str2 = "World"‘, you can concatenate them by using ‘str1 + " " + str2’, which will result in ‘"Hello World"‘.
Question: How do you concatenate strings?
Answer: Besides using the ‘+’ operator, strings in Python can also be concatenated using the ‘.join()’ method, which is particularly useful when concatenating a list of strings. For instance, ‘"".join([str1, str2])’ would concatenate ‘str1’ and ‘str2’ without any space. To add a space or any other separator, you could do ‘" ". join([str1, str2])’.
Question: How do you concatenate two strings?
Answer: To concatenate two strings, you can simply use the ‘+’ operator or the ‘.join()’ method as mentioned before. Additionally, you can use formatted strings (f-strings) as of Python 3.6, like ‘f"{str1} {str2}"‘, which is often more readable and efficient.
Question: How do you convert bytes to a string?
Answer: To convert bytes to a string in Python, you use the ‘.decode()’ method of bytes. Assuming ‘b = b"Hello World"‘, which is a bytes object, you can convert it to a string with ‘b.decode()’. By default, it uses UTF-8 encoding, but you can specify a different encoding if necessary.
Question: How do you convert data types in Python?
Answer: In Python, you can convert data types using built-in functions like ‘int()’, ‘float()’, ‘str()’, and ‘list()’. For example, to convert a string to an integer, you'd use ‘int("42")’, which would give you the integer ‘42’.
Question: How do you copy a file in Python?
Answer: To copy a file in Python, you can use the ‘shutil’ module which provides a utility function ‘shutil.copy(source, destination)’. This function copies the file from the source path to the destination path. If you want to copy the file's permission as well, you can use ‘shutil.copy2()’.
Question: How do you create a multidimensional list?
Answer: A multidimensional list in Python, often referred to as a list of lists, can be created by nesting lists inside a list. Here's an example of creating a 2D list, which is similar to a matrix:

Question: How do you create a read-only property in a class?
Answer: To create a read-only property in a class, you use the ‘property’ decorator on a method that returns a value. You do not define a setter for that property. Here's an example:
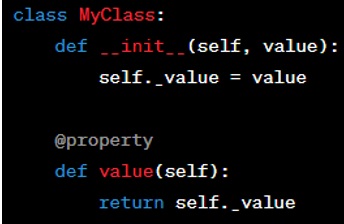
Question: How do you create a simple decorator?
Answer: A decorator in Python is a function that takes another function as an argument and extends its behavior without explicitly modifying it. Here's an example of a simple decorator that prints a message before and after the function call:
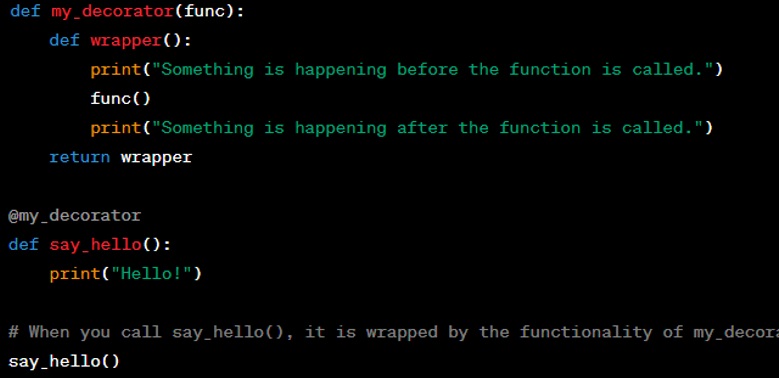
Question: How do you create a virtual environment in Python?
Answer: You can create a virtual environment in Python using the ‘venv’ module. Here's how you do it on the command line:
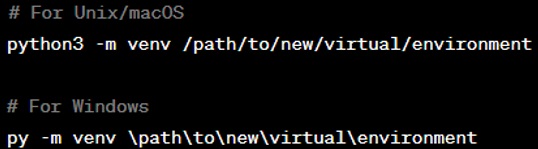
Question: How do you debug a Python program?
Answer: You can debug a Python program using various methods. The simplest is to insert ‘print’ statements to display values at different points in your code. For a more sophisticated approach, you can use the built-in ‘pdb’ module which provides an interactive debugging environment. Here's how you can use ‘pdb’:
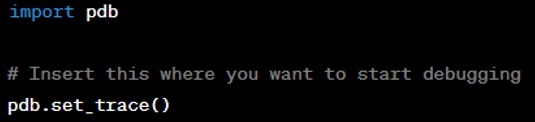
Question: How do you define a class in Python?
Answer: A class in Python is defined using the ‘class’ keyword followed by the class name and a colon. Here's a simple example:
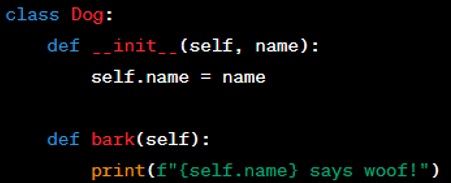
ReadioBook.com